用c语言在带头节点的单链表L中,删除所有值为x的结点,并释放其空间,假设值为x的结点不唯一,试编写算法以实现上述操作。
时间: 2024-10-09 10:02:28 浏览: 17
在C语言中,我们可以采用迭代的方式来遍历单链表并删除值为x的节点。这里是一个简单的算法步骤:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 删除值为x的节点
void deleteNodes(Node** head, int x) {
// 初始化新的头指针,表示无元素
Node* new_head = NULL;
Node* current = *head;
while (current != NULL) {
// 如果当前节点的数据等于x,跳过它并继续遍历
if (current->data == x) {
Node* temp = current;
current = current->next; // 移动到下一个节点
// 释放当前节点的空间
free(temp);
} else {
// 否则将当前节点添加到新链表中
new_head = (new_head == NULL) ? current : new_head->next;
current = current->next;
}
}
// 更新原始链表的头指针
*head = new_head;
}
// 测试函数
void printList(Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
// 创建示例链表
Node* list = createLinkedList(); // 这里需要你自己实现创建链表的函数
int value_to_remove = 5; // 要删除的值
deleteNodes(&list, value_to_remove);
// 打印更新后的链表
printList(list);
return 0;
}
```
在这个代码中,`deleteNodes` 函数接受链表的头指针和要删除的值 `x`,通过遍历链表,如果遇到值为 `x` 的节点就删除它。同时,一个新的链表 `new_head` 被构建,只包含那些值不为 `x` 的节点。
注意,你需要自己实现 `createLinkedList` 和相关的链表操作函数,如插入、创建等。
相关推荐
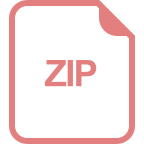
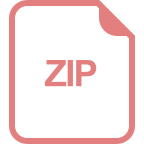
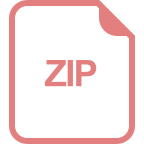






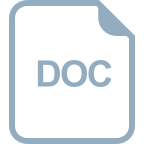







