使用C语言将一个二维数组存放在dat文件中,在MATLAB中打开这个dat文件存放在MATLAB编程语言使用二维数组
时间: 2024-12-13 18:25:39 浏览: 3
在C语言中,可以使用标准库函数`fopen`, `fwrite`, 和 `fclose` 来保存二维数组到dat文件。以下是一个简单的示例:
```c
#include <stdio.h>
// 假设我们有一个int类型的二维数组
int arr[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
void save_to_dat(char* filename, int arr[][4], int rows, int cols) {
FILE* file = fopen(filename, "wb"); // "wb"表示二进制写入模式
if (file == NULL) {
printf("Error opening the file.\n");
return;
}
// 写入行数和列数
fwrite(&rows, sizeof(int), 1, file);
fwrite(&cols, sizeof(int), 1, file);
// 写入数组内容
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
fwrite(&arr[i][j], sizeof(int), 1, file);
}
}
fclose(file);
}
// 在MATLAB中加载
void load_from_dat(char* filename, int** mat, int* row, int* col) {
FILE* file = fopen(filename, "rb"); // "rb"表示二进制读取模式
if (file == NULL) {
printf("Error opening the file.\n");
return;
}
*row = fread(row, sizeof(int), 1, file); // 读取行数
*col = fread(col, sizeof(int), 1, file); // 读取列数
int data_size = (*row) * (*col);
*mat = (int**)malloc(data_size * sizeof(int*));
for (int i = 0; i < *row; ++i) {
*mat[i] = (int*)malloc((*col) * sizeof(int)); // 分配每个行的内存
}
for (int i = 0; i < *row; ++i) {
for (int j = 0; j < *col; ++j) {
fread(*mat[i]+j, sizeof(int), 1, file); // 读取每个元素
}
}
fclose(file);
}
阅读全文
相关推荐
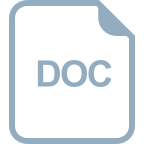
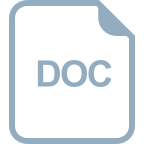
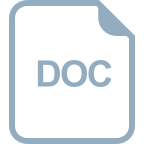
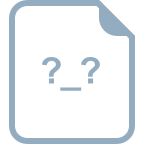
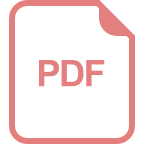
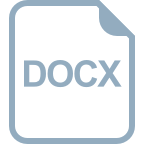
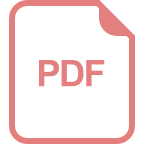
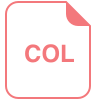
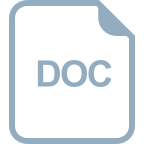
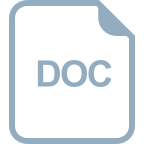
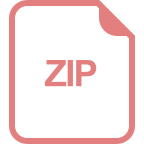
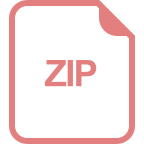
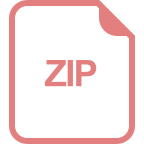
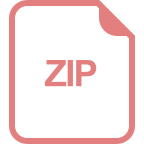
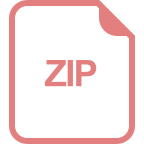
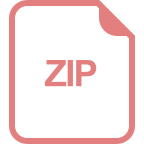
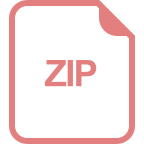