c++顺序表怎么建立具有5个元素的顺序表(定义类)
时间: 2024-10-04 10:02:31 浏览: 29
在C++中,我们可以定义一个顺序表(也称为线性表或动态数组)作为类来存储五个元素。下面是一个基础的顺序表实现示例,它包括了插入元素、获取元素以及打印列表的功能:
```cpp
#include <iostream>
// 顺序表类定义
class SequentialList {
private:
// 整型数组存储数据
int elements[5];
// 当前索引,表示已填充的元素个数
size_t currentIndex = 0;
public:
// 构造函数,初始化为空
SequentialList() {}
// 插入元素到列表末尾
void insert(int value) {
if (currentIndex < 5) {
elements[currentIndex] = value;
currentIndex++;
} else {
std::cout << "Error: List is full." << std::endl;
}
}
// 获取指定位置的元素
int get(size_t position) const {
if (position < currentIndex) {
return elements[position];
} else {
std::cout << "Error: Index out of bounds." << std::endl;
return -1; // 返回无效值
}
}
// 打印列表
void display() const {
for (size_t i = 0; i < currentIndex; i++) {
std::cout << "Element at index " << i << ": " << elements[i] << std::endl;
}
}
};
int main() {
// 创建顺序表实例并添加元素
SequentialList list;
list.insert(1);
list.insert(2);
list.insert(3);
list.insert(4);
list.insert(5); // 这里会提示错误,因为已满
// 显示列表
list.display();
return 0;
}
```
在这个例子中,我们限制了顺序表的大小为5,一旦达到这个限制,就不能再插入新的元素。
阅读全文
相关推荐
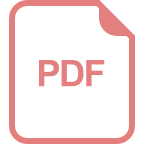
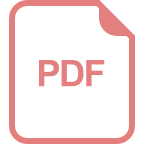
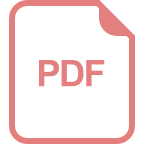
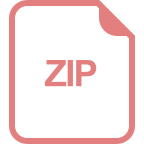
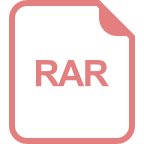
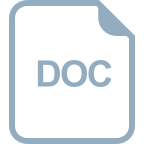
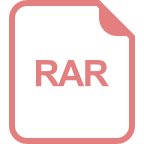
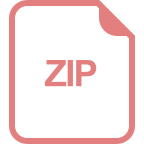
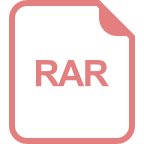
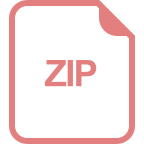
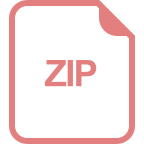
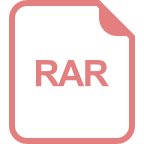
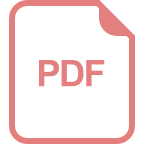
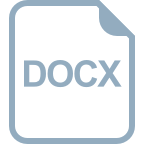
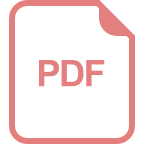
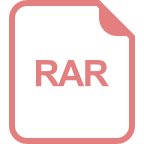