循环队列的实现c语言
时间: 2024-06-16 20:04:05 浏览: 100
循环队列是一种常见的队列实现方式,它可以有效地利用数组空间。在C语言中,可以通过以下步骤来实现循环队列:
1. 定义队列结构体:首先,我们需要定义一个队列结构体,包含队列的容量、头指针和尾指针等信息。例如:
```c
#define MAX_SIZE 10
typedef struct {
int data[MAX_SIZE];
int front; // 头指针
int rear; // 尾指针
} CircularQueue;
```
2. 初始化队列:在使用队列之前,需要对队列进行初始化,将头指针和尾指针都设置为-1。例如:
```c
void initQueue(CircularQueue *queue) {
queue->front = -1;
queue->rear = -1;
}
```
3. 判断队列是否为空:可以通过判断头指针和尾指针是否相等来确定队列是否为空。例如:
```c
int isEmpty(CircularQueue *queue) {
return queue-1;
}
```
4. 判断队列是否已满:可以通过判断尾指针的下一个位置是否等于头指针来确定队列是否已满。例如:
```c
int isFull(CircularQueue *queue) {
return (queue->rear + 1) % MAX_SIZE == queue->front;
}
```
5. 入队操作:将元素插入到队列的尾部,并更新尾指针的位置。例如:
```c
void enqueue(CircularQueue *queue, int value) {
if (isFull(queue)) {
printf("Queue is full.\n");
return;
}
if (isEmpty(queue)) {
queue->front = 0;
}
queue->rear = (queue->rear + 1) % MAX_SIZE;
queue->data[queue->rear] = value;
}
```
6. 出队操作:将队列头部的元素删除,并更新头指针的位置。例如:
```c
int dequeue(CircularQueue *queue) {
if (isEmpty(queue)) {
printf("Queue is empty.\n");
return -1;
}
int value = queue->data[queue->front];
if (queue->front == queue->rear) {
queue->front = -1;
queue->rear = -1;
} else {
queue->front = (queue->front + 1) % MAX_SIZE;
}
return value;
}
```
这样,我们就完成了循环队列的基本实现。
阅读全文
相关推荐
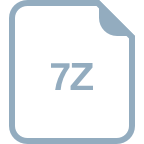
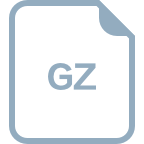
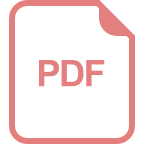
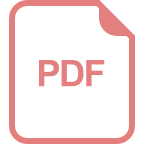
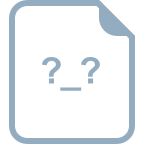
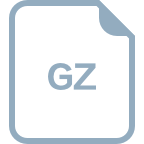
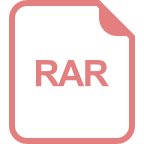
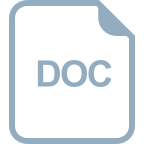
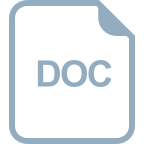
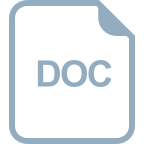
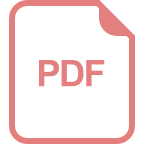