请用java来写1.摄氏度与华氏度的换算(华氏度=32+摄氏度*1.8)
时间: 2024-09-10 15:19:34 浏览: 38
当然可以。在Java中,你可以创建一个简单的函数来完成摄氏度到华氏度的转换。下面是一个例子:
```java
public class TemperatureConverter {
public static double celsiusToFahrenheit(double celsius) {
return (celsius * 1.8) + 32;
}
public static void main(String[] args) {
// 示例:将25摄氏度转换为华氏度
double celsius = 25;
double fahrenheit = celsiusToFahrenheit(celsius);
System.out.println("25摄氏度等于 " + fahrenheit + " 华氏度");
}
}
```
在这个代码片段中,`celsiusToFahrenheit` 方法接受摄氏温度作为输入,然后按照公式计算并返回相应的华氏温度。`main` 函数展示了如何调用这个方法。
相关问题
用Python写一段代码,内容是设计一个温度换算器,实现华氏度,摄氏度,开氏度之间的相互转换。其中,摄氏度=(华氏度-32)/1.8;华氏度=摄氏度*1.8+32;开氏度=摄氏度+273.15.
当然,下面是创建一个简单的温度转换器的Python代码:
```python
class TemperatureConverter:
def celsius_to_fahrenheit(self, celsius):
return (celsius * 1.8) + 32
def fahrenheit_to_celsius(self, fahrenheit):
return (fahrenheit - 32) / 1.8
def celsius_to_kelvin(self, celsius):
return celsius + 273.15
def kelvin_to_celsius(self, kelvin):
return kelvin - 273.15
# 使用示例
tc = TemperatureConverter()
temperature_input = float(input("请选择要转换的温度单位(例如: 华氏度 Celsius Kelvin): "))
conversion_type = input("请输入转换方向(例如: to_celsius from_fahrenheit): ").split()[0]
if conversion_type == "to":
unit = conversion_type[3:]
value = float(input(f"请输入{unit}值: "))
converted_temperature = getattr(tc, f"{unit}_to_{unit[0].lower()}", lambda x: x)(value)
else:
unit = conversion_type[6:]
value = float(input(f"请输入{unit}值: "))
converted_temperature = getattr(tc, f"{unit}_from_{unit[0].lower()}", lambda x: x)(value)
print(f"转换后的温度是: {converted_temperature} {unit}")
2.设计一个温度换算器,实现华氏度、摄氏度、开氏度之问的相互转换。其中,摄氏度 -(华氏度-32)/1.8;华氏度 二摄氏度x1.8+32;开氏度一摄氏度+273.15。
以下是使用Python语言编写的温度换算器代码:
```python
def celsius_to_fahrenheit(celsius):
fahrenheit = celsius * 1.8 + 32
return fahrenheit
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) / 1.8
return celsius
def celsius_to_kelvin(celsius):
kelvin = celsius + 273.15
return kelvin
def kelvin_to_celsius(kelvin):
celsius = kelvin - 273.15
return celsius
def fahrenheit_to_kelvin(fahrenheit):
celsius = fahrenheit_to_celsius(fahrenheit)
kelvin = celsius_to_kelvin(celsius)
return kelvin
def kelvin_to_fahrenheit(kelvin):
celsius = kelvin_to_celsius(kelvin)
fahrenheit = celsius_to_fahrenheit(celsius)
return fahrenheit
print("温度换算器\n")
while True:
print("请选择要转换的温度类型:")
print("1. 摄氏度")
print("2. 华氏度")
print("3. 开氏度")
print("4. 退出程序")
choice = int(input())
if choice == 1:
print("请输入摄氏度:")
celsius = float(input())
fahrenheit = celsius_to_fahrenheit(celsius)
kelvin = celsius_to_kelvin(celsius)
print("{:.2f}摄氏度 = {:.2f}华氏度 = {:.2f}开氏度\n".format(celsius, fahrenheit, kelvin))
elif choice == 2:
print("请输入华氏度:")
fahrenheit = float(input())
celsius = fahrenheit_to_celsius(fahrenheit)
kelvin = fahrenheit_to_kelvin(fahrenheit)
print("{:.2f}华氏度 = {:.2f}摄氏度 = {:.2f}开氏度\n".format(fahrenheit, celsius, kelvin))
elif choice == 3:
print("请输入开氏度:")
kelvin = float(input())
celsius = kelvin_to_celsius(kelvin)
fahrenheit = kelvin_to_fahrenheit(kelvin)
print("{:.2f}开氏度 = {:.2f}摄氏度 = {:.2f}华氏度\n".format(kelvin, celsius, fahrenheit))
elif choice == 4:
print("程序已退出。")
break
else:
print("输入有误,请重新选择。\n")
```
代码说明:
- 首先定义了六个函数,分别用于实现摄氏度与华氏度、摄氏度与开氏度、华氏度与开氏度之间的转换。
- 接着进入一个循环,显示菜单并等待用户输入选择。根据用户的选择调用相应的函数进行温度转换,并输出结果。
- 当用户选择“4”退出程序时,跳出循环并显示“程序已退出”。
阅读全文
相关推荐
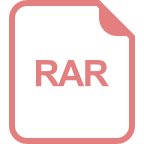
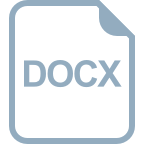











