使用FPGA实现UART串口通信代码
时间: 2024-04-11 21:26:30 浏览: 164
当然,下面是一个使用Verilog HDL编写的简单示例代码,用于在FPGA上实现UART串口通信:
```verilog
// 定义模块
module UART (
input wire clk,
input wire rst,
input wire uart_rx,
output wire uart_tx
);
// 定义参数
parameter BAUD_RATE = 9600;
parameter CLOCK_FREQ = 50000000;
// 定义局部变量
reg [3:0] bit_count;
reg [7:0] tx_data;
reg tx_busy;
reg [15:0] baud_divider;
reg [3:0] baud_count;
// 初始化模块
initial begin
bit_count = 0;
tx_data = 8'h00;
tx_busy = 0;
baud_divider = CLOCK_FREQ / BAUD_RATE;
baud_count = 0;
end
// 时钟分频器
always @(posedge clk) begin
if (rst) begin
baud_count <= 0;
end else begin
if (baud_count == baud_divider - 1) begin
baud_count <= 0;
end else begin
baud_count <= baud_count + 1;
end
end
end
// 发送数据
always @(posedge clk) begin
if (rst) begin
bit_count <= 0;
tx_busy <= 0;
end else begin
if (bit_count == 8) begin
bit_count <= 0;
tx_busy <= 1;
end else if (tx_busy == 1 && baud_count == baud_divider - 1) begin
bit_count <= bit_count + 1;
tx_data <= tx_data >> 1;
end
end
end
// 接收数据
always @(posedge clk) begin
if (rst) begin
// 处理接收数据
end else begin
// 处理接收数据
end
end
// 连接UART模块
assign uart_tx = tx_busy ? tx_data[0] : 1'b1;
endmodule
```
这段代码实现了一个简单的UART通信模块。其中,输入信号`uart_rx`为接收端口,输出信号`uart_tx`为发送端口。你可以根据需要进行修改和扩展,以满足你的具体应用需求。
阅读全文
相关推荐








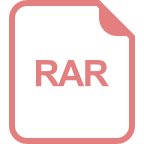







