设计模式装饰器java
时间: 2024-11-06 10:12:49 浏览: 4
设计模式中的装饰者模式(Decorator Pattern)是一种结构型模式,它允许动态地给一个对象添加新的功能,而无需修改其原有代码。在Java中,装饰者模式通常用于为对象添加更多责任或行为,而又不想对原始接口做任何改变。
在Java中,装饰者模式通过创建一个抽象组件类(Component),以及一系列装饰类(Decorator),每个装饰类都实现了该组件接口并包含对该组件的引用。当需要添加新功能时,我们创建一个新的装饰类实例,并将其作为组件实例的属性,从而扩展其行为。
举个例子:
```java
// 原始组件接口
public interface Component {
void operation();
}
// 装饰器接口
public abstract class Decorator implements Component {
protected Component component;
public Decorator(Component component) {
this.component = component;
}
@Override
public void operation() {
component.operation(); // 调用被装饰的组件
}
}
// 具体装饰类,如添加颜色
public class ColorDecorator extends Decorator {
public ColorDecorator(Component component) {
super(component);
}
// 添加额外的行为
@Override
public void operation() {
super.operation();
System.out.println("Adding color to the object.");
}
}
// 使用装饰者模式
public static void main(String[] args) {
Component original = new ComponentImpl(); // 原始组件实例
Component decorated = new ColorDecorator(original); // 创建装饰后的实例
decorated.operation(); // 输出原操作和颜色信息
}
```
阅读全文
相关推荐
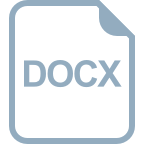
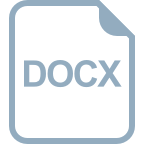
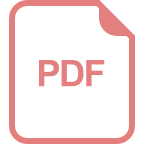
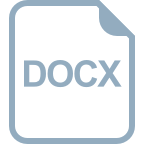
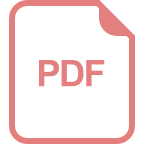
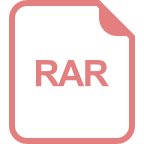
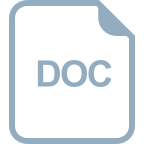
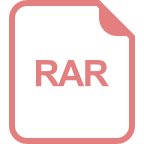
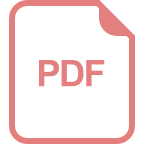
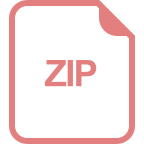
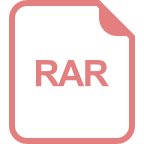
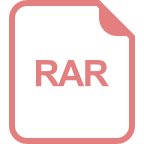
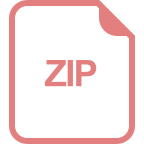
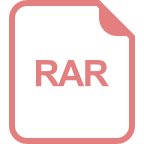
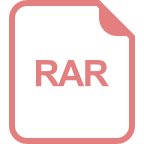
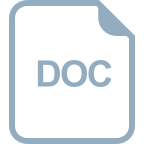
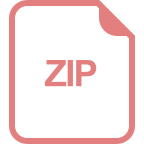
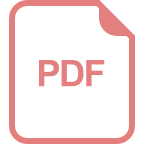
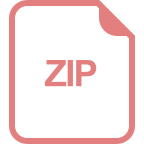