本题要求实现一个函数,实现大整数以整数形式存储。大整数按每4位保存在整数数组中,如果大整数位数不是4的倍数,则将保证低位都按4位一存。如“123456789”保存为1,2345,6789。大整数以字符串形式输入用C语言
时间: 2024-09-19 14:15:11 浏览: 80
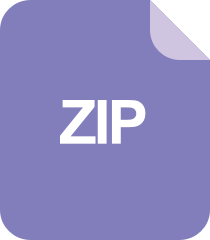
QT 的双精度数拆分和合并exe文件,win10 双精度浮点数拆为四个16位无符号整数以及将四个16位无符号整数组合
要实现在C语言中以整数形式存储大整数的功能,你可以创建一个名为`storeBigInteger`的函数,接受一个字符串作为输入,并按照每四位转换成一个整数的方式存储到一个整数数组中。以下是实现该功能的一个简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义一个结构体来辅助存储大整数的每一位
typedef struct {
int digit;
bool is_last; // 标记是否是四位数的最后一位
} BigDigit;
// 功能函数,用于处理大整数并存储到数组中
void storeBigInteger(char *str, int *result, size_t max_digits) {
// 初始化结果数组
BigDigit *bigInt = malloc(max_digits * sizeof(BigDigit));
memset(bigInt, 0, max_digits * sizeof(BigDigit));
size_t i = 0;
while (i < strlen(str)) {
// 每次处理四位字符
char四位[5];
strncpy(four, str + i, 4);
four[4] = '\0'; // 如果不足四位,填充'\0'
int num = atoi(four); // 转换四位字符为整数
// 存储每一位数字
bigInt[i / 4].digit = num;
if (strlen(four) == 4 || i == strlen(str) - 1) { // 最后一位或者已经结束
bigInt[i / 4].is_last = true;
} else {
bigInt[i / 4].is_last = false;
}
i += 4;
}
// 把大整数转换回数组表示的形式
for (size_t j = 0; j < max_digits; j++) {
if (bigInt[j].is_last) {
result[j] = bigInt[j].digit;
} else {
result[j] = bigInt[j].digit * pow(10, (max_digits - 1 - j) * 4);
}
}
free(bigInt);
}
int main() {
char str[] = "123456789";
int result[10]; // 假设最大可能有10位数字
storeBigInteger(str, result, sizeof(result) / sizeof(result[0]));
// 打印结果
for (int i = 0; i < sizeof(result) / sizeof(result[0]); i++) {
printf("%d", result[i]);
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐
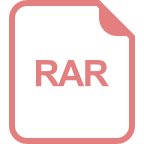















