、使用系统文件IO实现链表的读写操作 要求: 封装4个函数,分别是创建链表,遍历链表, 写链表,读链表 先写一个程序创建链表,然后写入文件 然后再写一个程序读链表,遍历链表用
时间: 2024-08-16 09:03:16 浏览: 37
首先,我们需要定义一个链表节点结构体,然后实现创建链表、遍历链表、写链表和读链表的函数。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
// 创建链表
Node *create_list(int n) {
Node *head = NULL, *tail = NULL;
for (int i = 0; i < n; i++) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->data = i + 1;
new_node->next = NULL;
if (head == NULL) {
head = new_node;
tail = new_node;
} else {
tail->next = new_node;
tail = new_node;
}
}
return head;
}
// 遍历链表
void traverse_list(Node *head) {
Node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("
");
}
// 写链表到文件
void write_list_to_file(Node *head, const char *filename) {
FILE *file = fopen(filename, "w");
if (file == NULL) {
printf("无法打开文件
");
return;
}
Node *current = head;
while (current != NULL) {
fprintf(file, "%d ", current->data);
current = current->next;
}
fclose(file);
}
// 从文件中读取链表
Node *read_list_from_file(const char *filename) {
FILE *file = fopen(filename, "r");
if (file == NULL) {
printf("无法打开文件
");
return NULL;
}
Node *head = NULL, *tail = NULL;
int data;
while (fscanf(file, "%d", &data) == 1) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->data = data;
new_node->next = NULL;
if (head == NULL) {
head = new_node;
tail = new_node;
} else {
tail->next = new_node;
tail = new_node;
}
}
fclose(file);
return head;
}
int main() {
int n = 5;
Node *head = create_list(n);
traverse_list(head);
write_list_to_file(head, "list.txt");
Node *read_head = read_list_from_file("list.txt");
traverse_list(read_head);
return 0;
}
```
这个程序首先创建一个包含5个节点的链表,然后将其写入名为"list.txt"的文件。接着,它从该文件中读取链表并遍历打印出来。
阅读全文
相关推荐
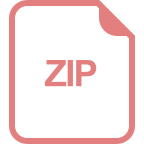
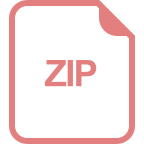
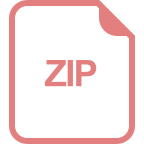















