java http form请求代码示例
时间: 2023-09-04 08:13:11 浏览: 50
以下是Java中使用HttpURLConnection发送Form请求的示例代码:
```java
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
public class FormRequestDemo {
public static void main(String[] args) throws IOException {
String url = "http://example.com/api/user";
String formData = "username=johndoe&password=123456";
byte[] formDataBytes = formData.getBytes(StandardCharsets.UTF_8);
URL apiUrl = new URL(url);
HttpURLConnection connection = (HttpURLConnection) apiUrl.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
connection.setRequestProperty("Content-Length", Integer.toString(formDataBytes.length));
connection.setDoOutput(true);
DataOutputStream outputStream = new DataOutputStream(connection.getOutputStream());
outputStream.write(formDataBytes);
outputStream.flush();
outputStream.close();
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
}
}
```
代码中,`url`变量表示请求的URL地址,`formData`变量表示提交的表单数据。首先将表单数据转换为字节数组,然后设置请求头信息,包括Content-Type和Content-Length。设置完请求头之后,将表单数据写入到请求体中,然后发送请求。最后,通过getResponseCode()方法获取响应码。
注意,这里的请求方式是POST,如果是GET请求,可以将请求数据拼接到URL后面,例如:
```java
String url = "http://example.com/api/user?username=johndoe&password=123456";
URL apiUrl = new URL(url);
HttpURLConnection connection = (HttpURLConnection) apiUrl.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
```
这里的请求方式是GET,请求数据通过URL的参数传递。在实际开发中,需要根据具体的接口文档和要求来选择合适的请求方式和数据传输方式。
相关推荐
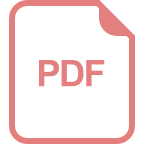
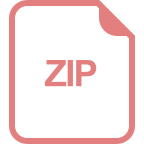














