用c++编写 给定一棵非空二叉树,请在该二叉树中查找给定值的结点(唯一),并输出其祖先(从根结点到此结点经过的点)结点的元素值;若不存在,则输出0。
时间: 2024-11-30 10:17:04 浏览: 13
在C++中,我们可以使用递归的方式来解决这个问题。首先,我们需要定义一个二叉树节点结构体,并实现一个函数来搜索目标节点及其祖先。这里是一个简单的解决方案:
```cpp
#include <iostream>
using namespace std;
// 定义二叉树节点
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 辅助函数,用于找到路径中的每个节点值
void findPath(TreeNode* root, vector<int>& ancestors, TreeNode* target, int path[], int& idx) {
if (!root) return;
// 如果找到了目标节点,将其添加到祖先列表中并返回
if (root == target) {
for (int i = idx; i > 0; --i) {
ancestors.push_back(path[i]);
}
return;
}
// 递归搜索左子树和右子树
path[idx++] = root->val;
findPath(root->left, ancestors, target, path, idx);
findPath(root->right, ancestors, target, path, idx);
// 当前节点不是目标,回溯并移除路径中的最后一个值
idx--;
}
// 主函数,输入树和目标值,输出祖先节点值
void searchAncestors(TreeNode* root, int target) {
if (!root) return;
int path[50], idx = 0;
vector<int> ancestors;
findPath(root, ancestors, target, path, idx);
// 输出祖先节点值(从根开始)
for (const auto& ancestor : ancestors)
cout << ancestor << " ";
cout << endl;
if (ancestors.empty()) cout << 0 << endl; // 如果未找到目标节点,输出0
}
int main() {
// 假设你已经有了一个二叉树实例,这里仅示例如何调用这个函数
TreeNode* tree = ...; // 初始化你的二叉树
int targetValue = 10;
searchAncestors(tree, targetValue);
return 0;
}
```
在这个程序中,`searchAncestors` 函数接受二叉树的根节点、目标值作为输入。它首先创建一个临时数组 `path` 来存储搜索过程中的节点值,然后递归地检查每个节点,如果找到目标,就将路径中的所有祖先节点值加入到 `ancestors` 列表中。如果没有找到目标节点,最后会输出 0。
阅读全文
相关推荐
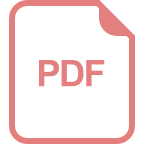
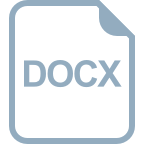
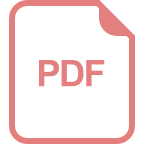
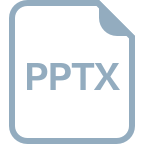
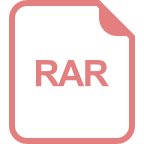
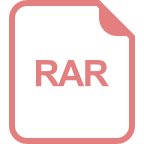
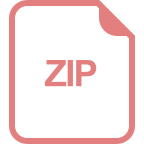
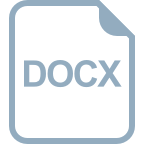
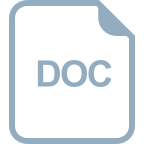
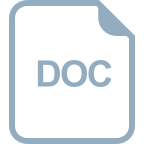
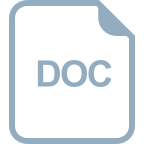
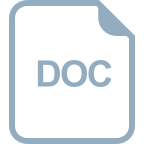

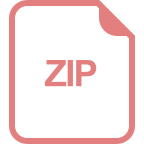
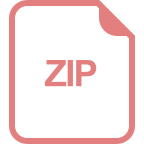
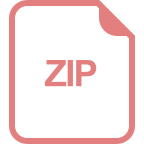