给定一个8位数12345678,输入要交换的两个数位,输出交换后的数字。 注:第1数位、第2数位、第3数位 ... 分别为:个、十、百 ...c语言
时间: 2024-09-29 21:03:57 浏览: 9
你可以使用C语言中的结构体和指针来实现这个功能。首先定义一个结构体表示八位数,然后编写函数接受用户输入并交换指定位置的数字。下面是一个简单的示例:
```c
#include <stdio.h>
// 定义一个结构体代表8位数
typedef struct {
unsigned char digit[8];
} EightBitNumber;
// 函数用于交换结构体中两个指定位置的数字
void swap_digits(EightBitNumber* num, int pos1, int pos2) {
// 检查索引是否有效
if (pos1 >= 0 && pos1 < 8 && pos2 >= 0 && pos2 < 8) {
unsigned char temp = num->digit[pos1]; // 临时存储第一个位置的数字
num->digit[pos1] = num->digit[pos2]; // 将第二个位置的数字放到第一个位置
num->digit[pos2] = temp; // 将临时存储的数字放到第二个位置
} else {
printf("Invalid index.\n");
}
}
int main() {
EightBitNumber number;
number.digit[0] = 1; // 初始化个位
number.digit[1] = 2;
number.digit[2] = 3;
// ... 以此类推,初始化其他位
int pos1, pos2;
printf("Enter the positions to swap (separated by space): ");
scanf("%d %d", &pos1, &pos2);
swap_digits(&number, pos1 - 1, pos2 - 1); // 减1是因为数组索引从0开始
printf("After swapping, the number is: ");
for (int i = 0; i < 8; i++) {
printf("%d", number.digit[i]);
}
printf("\n");
return 0;
}
```
在这个程序中,用户输入两个位置索引,`swap_digits`函数会交换这两个位置的数字。注意索引是从0开始计数的,所以在输入时需要减去1。
相关推荐
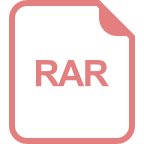
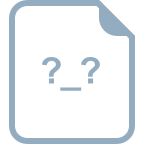
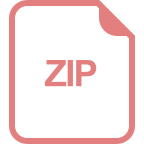














