python修改mysql表中数据并显示
时间: 2024-09-27 13:07:27 浏览: 35
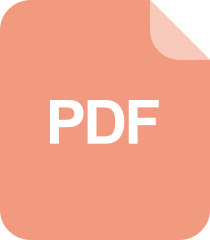
Python爬取数据并写入MySQL数据库的实例
在Python中,我们可以使用`pymysql`库或更现代的`mysql-connector-python`库来连接MySQL数据库,并使用SQL语句来更新表中的数据。这里是一个基本的例子:
首先,你需要安装必要的库(如果尚未安装):
```bash
pip install pymysql mysql-connector-python
```
然后,你可以使用以下步骤来更新数据并显示结果:
1. 连接到MySQL数据库:
```python
import pymysql
# 数据库配置信息
db_config = {
'host': 'your_host',
'user': 'your_username',
'password': 'your_password',
'database': 'your_database'
}
# 创建连接
connection = pymysql.connect(**db_config)
try:
with connection.cursor() as cursor:
# 更新数据示例(假设有一个名为'table_name'的表和你要更新的字段名)
update_query = "UPDATE table_name SET column_to_update = 'new_value' WHERE condition" # 条件根据实际情况填写
cursor.execute(update_query)
connection.commit() # 提交事务
except pymysql.Error as e:
print(f"Error: {e}")
finally:
if connection:
connection.close()
print("Database connection closed.")
```
2. 如果你想查询更新后的结果并显示,可以添加一个新的查询语句:
```python
select_query = "SELECT * FROM table_name WHERE condition" # 同样填入适当的条件
cursor.execute(select_query)
updated_rows = cursor.fetchall()
for row in updated_rows:
print(row) # 打印获取到的数据行
```
记得替换上述代码中的占位符(如`your_host`、`your_username`等)为实际的数据库连接信息。
阅读全文
相关推荐
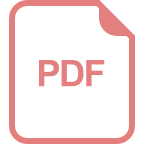
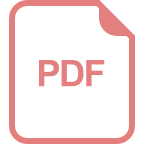
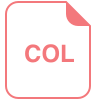
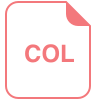













