3维空间的两个点p1=(x1,x2,...xk)和p2=(y1,y2,...yk)的欧几里得距离定义为d(p1,p2)=(∑(yi-xi)²)二分之一次方,蛮力法求3维空间最近的点对,写出C++代码
时间: 2024-10-25 21:13:29 浏览: 31
在三维空间中,给定一组点,可以使用蛮力法(也称为暴力法或穷举法)计算每一对点之间的欧氏距离,并找出最小的距离。下面是一个简单的C++代码示例,它会创建一个二维数组存储所有点,然后遍历数组寻找最近的一对点:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
#include <limits.h> // 使用INT_MAX作为初始最大值
using namespace std;
const double INF = INT_MAX;
// 定义三维点结构体
struct Point3D {
double x, y, z;
};
// 计算两点之间的欧氏距离
double distance(const Point3D &p1, const Point3D &p2) {
return sqrt(pow(p2.x - p1.x, 2) + pow(p2.y - p1.y, 2) + pow(p2.z - p1.z, 2));
}
// 蛮力法寻找最近的点对
pair<Point3D, Point3D> findNearestPair(vector<Point3D> points) {
pair<Point3D, Point3D> nearest Pair(points[0], points[0]); // 初始化为第一个点
for (size_t i = 1; i < points.size(); ++i) {
for (size_t j = 0; j < i; ++j) {
double currentDistance = distance(points[i], points[j]);
if (currentDistance < nearest.second.distance) {
nearest.first = points[i];
nearest.second = points[j];
}
}
}
return nearest;
}
int main() {
vector<Point3D> points = { // 你可以在这里输入或生成你的点
{1, 2, 3}, {4, 5, 6}, {7, 8, 9}, {10, 11, 12}
};
pair<Point3D, Point3D> nearestPair = findNearestPair(points);
cout << "The nearest pair is (" << nearestPair.first.x << ", "
<< nearestPair.first.y << ", " << nearestPair.first.z << ") and ("
<< nearestPair.second.x << ", " << nearestPair.second.y << ", "
<< nearestPair.second.z << ").\n";
return 0;
}
```
这个程序首先初始化最近的一对点为第一个点,然后通过两层循环遍历所有的点对,比较它们之间的距离,如果发现新的更近的点对,就更新结果。
阅读全文
相关推荐
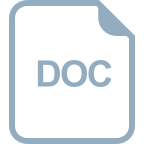
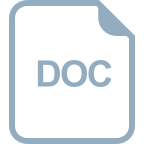
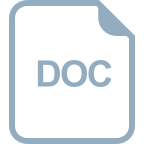
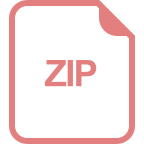
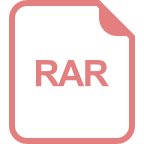
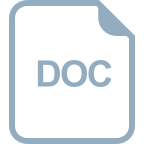
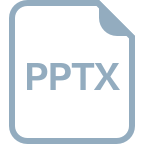
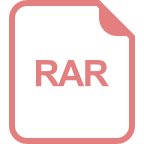
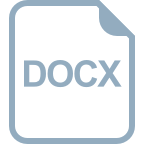
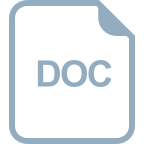
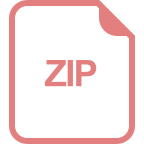
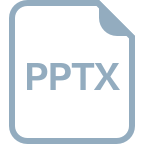
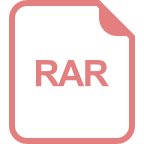
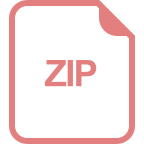
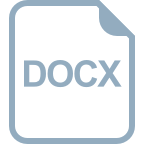
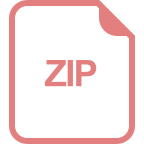
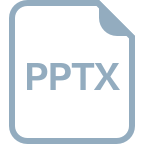
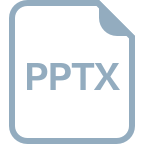