TRT_LOGGER = trt.Logger(trt.Logger.INFO) AttributeError: module 'tensorrt' has no attribute 'Logger'
时间: 2023-12-05 13:41:11 浏览: 345
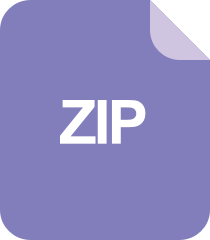
torch2trt:易于使用的PyTorch到TensorRT转换器
这个错误通常是由于TensorRT版本不兼容或未正确安装所致。请确保您已正确安装TensorRT并使用正确的版本。您可以尝试使用以下命令检查TensorRT版本:
```shell
dpkg -l | grep nvinfer
```
如果您使用的是TensorRT 7,则应该使用以下代码创建Logger:
```python
TRT_LOGGER = trt.Logger(trt.Logger.INFO)
```
如果您使用的是TensorRT 6或更早版本,则应该使用以下代码创建Logger:
```python
TRT_LOGGER = trt.Logger(trt.Logger.VERBOSE)
```
阅读全文
相关推荐
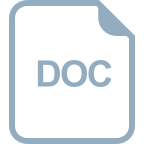
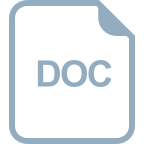
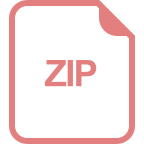
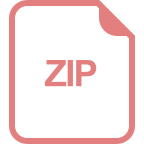
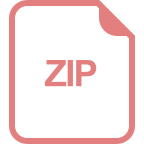












