java轮播图(完整版)需求: 1每隔一秒钟切换一个图片,文字及背景颜色。 2 小圆点按顺序点亮 3 图片播放到最后一张自动复原从头播放 4当点击左右的按钮,可以切换轮播图 5 鼠标经过图片停止播放,鼠标移开继续播放
时间: 2024-12-23 22:14:08 浏览: 16
在Java中实现一个轮播图功能,通常会结合Swing、JavaFX或者HTML+CSS+JavaScript(如JSF框架)。这里我会给出一个基于Swing库的基本示例,因为Swing是Java的标准GUI工具包,适合用于桌面应用。为了简化,我将提供关键部分的代码片段。
首先,创建一个JFrame作为容器:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Carousel extends JFrame {
private JLabel[] images;
private JButton prevBtn, nextBtn;
private Timer timer;
private int currentIndex = 0;
public Carousel() {
// 初始化组件和设置
initComponents();
}
// ...其他构造函数和初始化方法
private void initComponents() {
// 创建图像标签数组
images = new JLabel[3]; // 假设有三张图片
// 添加小圆点指示器
JToolBar toolbar = new JToolBar();
for (int i = 0; i < images.length; i++) {
JButton dotButton = new JButton();
dotButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (!timer.isRunning()) {
changeSlide(dotButton.getText().equals("1"));
}
}
});
if (i == currentIndex) {
dotButton.setText("1");
dotButton.setBackground(Color.YELLOW);
} else {
dotButton.setText(Integer.toString(i + 1));
}
toolbar.add(dotButton);
}
// 添加图片和事件监听
add(images[currentIndex], BorderLayout.CENTER);
prevBtn = new JButton("prev");
nextBtn = new JButton("next");
prevBtn.addActionListener(this::prevSlide);
nextBtn.addActionListener(this::nextSlide);
JPanel buttonPanel = new JPanel(new FlowLayout());
buttonPanel.add(prevBtn);
buttonPanel.add(nextBtn);
add(buttonPanel, BorderLayout.PAGE_START);
// 设置定时器
timer = new Timer(1000, this);
timer.start();
}
private void changeSlide(boolean first) {
if (first) {
currentIndex = 0;
} else {
currentIndex++;
if (currentIndex >= images.length) {
currentIndex = 0;
}
}
updateSlide();
}
private void prevSlide(ActionEvent e) {
if (currentIndex > 0) {
changeSlide(false);
}
}
private void nextSlide(ActionEvent e) {
if (currentIndex < images.length - 1) {
changeSlide(true);
}
}
private void updateSlide() {
images[currentIndex].setIcon(images[currentIndex].getImage()); // 这里假设图片是ImageIcon类型
// 更新背景颜色,如果需要动态改变的话
}
// 更多Swing的窗口管理方法...
}
```
这个示例实现了基本的需求,包括定时切换图片、小圆点指示器和按钮控制。对于鼠标悬停暂停和移开继续播放,可以在`updateSlide()`方法中添加鼠标事件监听,并根据`MouseListener`的`mouseMoved()`和`mouseExited()`方法来控制定时器。
阅读全文
相关推荐
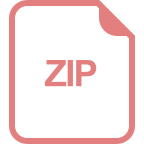
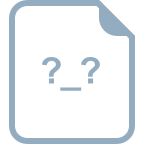
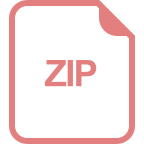















