hmacsha256加密算法 c语言
时间: 2023-09-07 13:01:50 浏览: 275
HmacSha256是一种基于SHA-256(Secure Hash Algorithm 256-bit)算法的消息鉴别码算法。在C语言中,可以使用OpenSSL库来实现HmacSha256加密算法。
首先,我们需要包含OpenSSL库的头文件和链接静态库文件。在C代码中,可以使用以下指令进行包含:
#include <openssl/hmac.h>
```
gcc -o example example.c -lcrypto
```
接下来,我们可以定义一个函数来实现HmacSha256加密算法:
```
#include <stdio.h>
#include <string.h>
#include <openssl/hmac.h>
void hmac_sha256(const unsigned char *key, int key_len, const unsigned char *data, int data_len, unsigned char *digest) {
HMAC_CTX *hmac_ctx;
unsigned int sha256_len;
hmac_ctx = HMAC_CTX_new();
HMAC_Init_ex(hmac_ctx, key, key_len, EVP_sha256(), NULL);
HMAC_Update(hmac_ctx, data, data_len);
HMAC_Final(hmac_ctx, digest, &sha256_len);
HMAC_CTX_free(hmac_ctx);
}
int main() {
unsigned char key[] = "secret_key";
unsigned char data[] = "message";
int key_len = strlen(key);
int data_len = strlen(data);
unsigned char digest[EVP_MAX_MD_SIZE];
hmac_sha256(key, key_len, data, data_len, digest);
printf("HMAC-SHA256 digest: ");
for (int i = 0; i < SHA256_DIGEST_LENGTH; i++) {
printf("%02x", digest[i]);
}
printf("\n");
return 0;
}
```
在main函数中,我们使用一个密钥key和一个消息data来进行HmacSha256加密,结果存储在digest数组中。输出结果是一个64字节的摘要。
编译并运行这段代码,将会输出HMAC-SHA256摘要结果。
这就是在C语言中实现HmacSha256加密算法的简单示例。
阅读全文
相关推荐

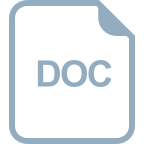
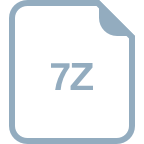
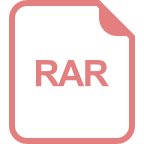
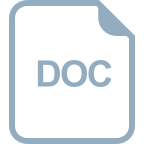
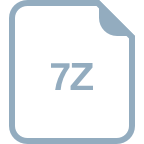
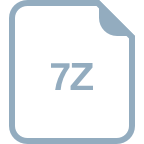
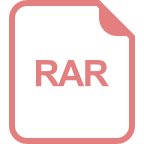
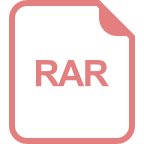
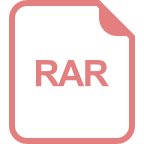
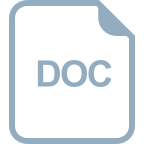
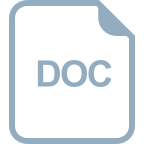
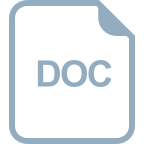
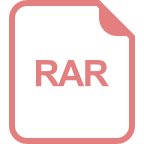