数据结构使用双向循环链表表示图书管理系统代码,能增删查改
时间: 2024-10-27 20:05:38 浏览: 12
在数据结构中,使用双向循环链表(Doubly Linked List)来设计图书管理系统可以提供高效的操作能力。这是一种链接节点的数据结构,每个节点包含书的信息(如书籍ID、标题、作者等),以及指向前一个和后一个节点的引用。
以下是简单的图书管理系统的代码示例(这里以Python语言为例):
```python
class Node:
def __init__(self, book_id, title, author):
self.book_id = book_id
self.title = title
self.author = author
self.prev = None # 指向前一个节点
self.next = None # 指向后一个节点
class LibrarySystem:
def __init__(self):
self.head = None
self.tail = None
def add_book(self, book_id, title, author):
new_node = Node(book_id, title, author)
if not self.head:
self.head = self.tail = new_node
else:
new_node.prev = self.tail
self.tail.next = new_node
self.tail = new_node
def remove_book(self, book_id):
current = self.head
while current:
if current.book_id == book_id:
if current.prev:
current.prev.next = current.next
elif self.head == current: # 头结点被删除的情况
self.head = current.next
if current.next:
current.next.prev = current.prev
else: # 尾结点被删除的情况
self.tail = current.prev
return True
current = current.next
return False # 未找到指定书目
def search_book(self, book_id):
current = self.head
while current:
if current.book_id == book_id:
return True
current = current.next
return False # 未找到指定书目
def update_book(self, old_book_id, new_title, new_author):
current = self.head
while current:
if current.book_id == old_book_id:
current.title = new_title
current.author = new_author
break
current = current.next
# 使用示例
library = LibrarySystem()
library.add_book(1, "Book1", "Author1")
# ... 其他操作 ...
```
阅读全文
相关推荐
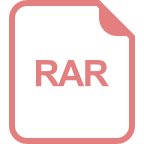
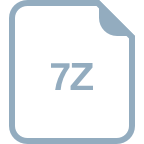
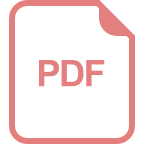
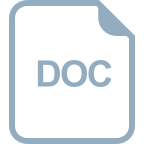
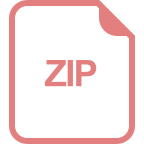
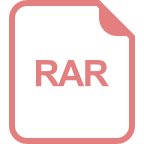
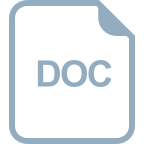
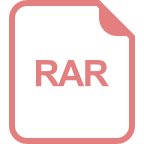
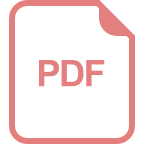
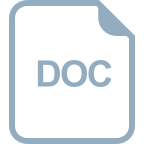
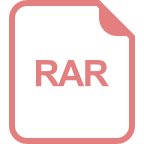
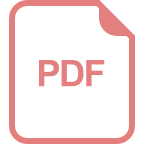
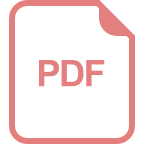
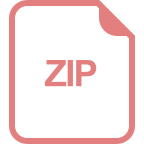