c++景区旅游信息管理
时间: 2023-12-22 18:29:11 浏览: 101
C++实现景区旅游信息管理可以通过使用有向图来表示导游线路图,并通过遍历景点来制定旅游景点导游线路策略。以下是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义景点结构体
struct Spot {
string name;
vector<int> nextSpots; // 存储下一个可到达的景点的索引
};
// 定义景区旅游信息管理类
class TourismManagement {
private:
vector<Spot> spots; // 存储所有景点的数组
public:
// 添加景点
void addSpot(const string& name) {
Spot spot;
spot.name = name;
spots.push_back(spot);
}
// 添加导游线路
void addRoute(int from, int to) {
spots[from].nextSpots.push_back(to);
}
// 判断导游线路图中是否有回路
bool hasCycle() {
vector<bool> visited(spots.size(), false); // 记录每个景点是否被访问过
vector<bool> onPath(spots.size(), false); // 记录当前遍历路径上的景点
for (int i = 0; i < spots.size(); i++) {
if (dfs(i, visited, onPath)) {
return true;
}
}
return false;
}
private:
// 深度优先搜索遍历
bool dfs(int spotIndex, vector<bool>& visited, vector<bool>& onPath) {
visited[spotIndex] = true;
onPath[spotIndex] = true;
for (int nextSpotIndex : spots[spotIndex].nextSpots) {
if (!visited[nextSpotIndex] && dfs(nextSpotIndex, visited, onPath)) {
return true;
} else if (onPath[nextSpotIndex]) {
return true;
}
}
onPath[spotIndex] = false;
return false;
}
};
int main() {
TourismManagement tm;
// 添加景点
tm.addSpot("A");
tm.addSpot("B");
tm.addSpot("C");
tm.addSpot("D");
// 添加导游线路
tm.addRoute(0, 1);
tm.addRoute(1, 2);
tm.addRoute(2, 3);
tm.addRoute(3, 0);
// 判断导游线路图中是否有回路
bool hasCycle = tm.hasCycle();
cout << "导游线路图中是否有回路: " << (hasCycle ? "是" : "否") << endl;
return 0;
}
```
这个示例中,我们首先定义了一个`Spot`结构体来表示每个景点,其中包含景点的名称和一个存储下一个可到达的景点的索引的向量。然后,我们定义了一个`TourismManagement`类来管理景区旅游信息,其中包含添加景点和添加导游线路的方法。最后,我们使用深度优先搜索算法来判断导游线路图中是否有回路。
阅读全文
相关推荐
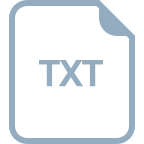
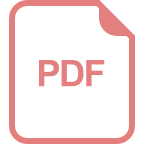
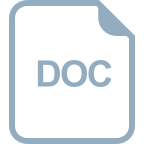
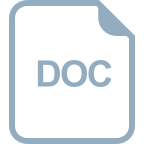

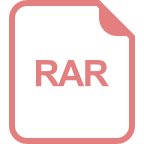
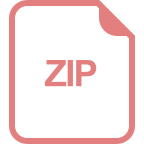
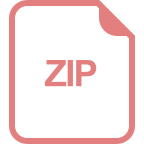
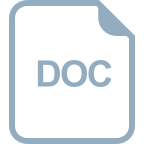
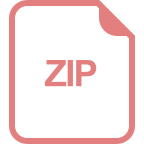
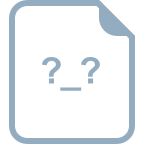
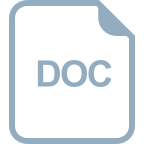



