编写一段C#代码,实现角度前方交会:
时间: 2024-12-25 15:25:08 浏览: 16
在C#中,要实现两点之间的角度前方交会,我们可以创建一个静态方法,接受两个点的坐标作为输入,并计算它们之间的线段的角度。假设我们有两个Point类,可以这样做:
```csharp
using System;
public class Point {
public double X { get; set; }
public double Y { get; set; }
// 简单构造函数
public Point(double x, double y) {
X = x;
Y = y;
}
}
public static class LineIntersection {
// 计算两点间线段与x轴正方向之间的夹角(逆时针为正)
public static double GetAngle(Point p1, Point p2) {
if (p1.Y == p2.Y) return 0; // 平行于x轴,无角度
double slope = (p2.Y - p1.Y) / (p2.X - p1.X); // 斜率
return Math.Atan(slope); // 使用反正切函数获取角度
}
}
// 示例使用
class Program {
static void Main(string[] args) {
Point pointA = new Point(0, 0);
Point pointB = new Point(5, 3);
double angle = LineIntersection.GetAngle(pointA, pointB);
Console.WriteLine($"角度: {angle} 弧度 (注意这是相对于X轴正方向的角度)");
// 转换为度数并打印
double degrees = angle * 180 / Math.PI;
Console.WriteLine($"角度: {degrees} 度");
}
}
```
在这个例子中,我们首先定义了两个点,然后通过`GetAngle`方法计算这两个点之间连线与水平方向的角度。注意,C#中弧度制是默认单位。
阅读全文
相关推荐




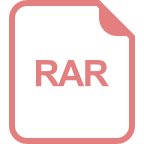
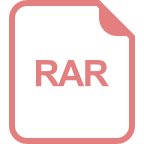

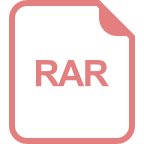


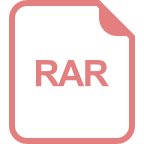





