使用Scrapy对小红书热点的前50条信息进行爬取 并且必须使用yield item语句 每一行代码都要详细注释 并详细给出要怎么展示爬出的内容
时间: 2024-10-11 10:12:19 浏览: 83
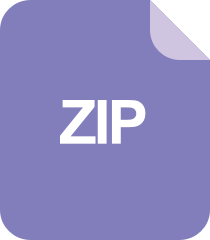
使用scrapy框架 对房天下的房源信息进行爬取数据

使用Scrapy框架爬取小红书的热点内容并提取前50条信息需要编写一个基本的Spider,并利用yield item语句来逐行返回爬取到的数据。这里我会提供一个简单的示例,首先确保已经安装了必要的库:
```python
# 首先安装必要的库(如果你还没有安装)
# pip install scrapy requests lxml beautifulsoup4
import scrapy
from bs4 import BeautifulSoup
from scrapy.http import Request
class XiaohongshuHotSpiders(scrapy.Spider):
name = 'xiaohongshu_hot'
# 设置起始URL和请求头,模拟真实浏览器访问
start_urls = ['https://www.xiaohongshu.com/explore']
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
def parse(self, response):
"""
解析HTML响应,获取每个帖子的基本信息
"""
# 解析HTML,查找包含帖子数据的部分(这通常是一个列表或者分页容器)
soup = BeautifulSoup(response.text, 'lxml')
hot_posts_list = soup.find('div', {'class': 'post-grid__list'})
if hot_posts_list is not None:
# 对于每一条热门帖子...
for post in hot_posts_list.find_all('li', {'class': '_3qQVU'}): # 选择帖子元素
item = {} # 创建空字典用于存储item
# 提取标题(假设在h3标签内)
title = post.find('h3', {'class': '_2iDmM'}).text
item['title'] = title.strip() # 去除首尾空白
# 提取作者信息(假设在p标签内)
author_info = post.find('a', {'class': '_3cYRz'})
if author_info:
author_name = author_info.text
item['author'] = author_name.strip()
else:
item['author'] = None # 如果找不到,设置为空
# 提取发布日期(假设在span标签内,注意实际网站结构可能会变化)
date = post.find('time', {'class': '_3tIYv'}).text
item['date'] = date.strip()
# 提供链接(同理,这里假定链接在'a'标签内)
link = post.find('a')['href']
item['link'] = 'https://www.xiaohongshu.com/' + link # 构建完整的URL
# 使用yield关键字返回item,告诉Scrapy将这个item暂存并处理完后再继续下一个
yield item
# 当达到50个帖子时停止爬取,防止过度抓取
if len(item.items()) >= 50:
break
# 为了继续抓取下一页,找到分页链接并发送新请求
next_page_link = response.css('a.next::attr(href)').get()
if next_page_link:
yield Request(url=response.urljoin(next_page_link), callback=self.parse, headers=self.headers)
else:
# 如果找不到帖子列表,打印一条消息并结束爬虫
print("No posts found on this page.")
def closed(self, reason):
"""
爬虫关闭时的回调函数
"""
print(f"Spider closed with reason {reason}")
```
当你运行这个Spider时,它会按照上述逻辑爬取小红书的热门帖子。爬取结果将以字典形式保存,每一项代表一条帖子的信息,如标题、作者、发布时间和链接。如果遇到超过50条的限制,它会在第50条之后停止。
展示爬取内容的方式取决于你的需求,你可以将其存储在一个CSV文件、数据库或者直接通过程序进行分析。例如:
```python
for post in self.crawled_data:
print(f"{post['title']} - {post['author']} ({post['date']}):\n{post['link']}\n")
```
这只是一个基础的示例,实际的小红书页面结构可能会有所不同,你需要根据实际HTML调整解析部分。同时,遵守网站的Robots协议,不要对服务器造成过大压力。
阅读全文
相关推荐
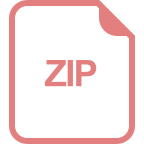


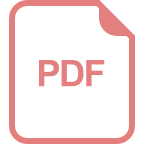
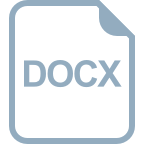
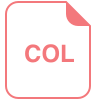
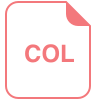
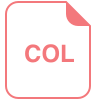
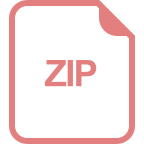
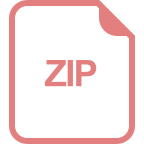
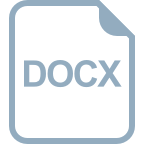