acc_vec * dt中acc_vec是一个1X3的行向量,dt是一个标量,如何使acc_vec*dt变成1X1304的行向量,给出matlab代码
时间: 2024-10-24 09:14:41 浏览: 28
为了将一个1x3的行向量 `acc_vec` 乘以标量 `dt` 后得到一个1x1304的行向量,你需要将 `acc_vec` 重复应用1304次。你可以使用循环结构(比如for循环)或数组操作来实现这个目标。以下是使用循环的一个例子:
```matlab
dt = yourScalarValue; % 替换为实际的dt值
target_length = 1304;
result = zeros(1, target_length); % 初始化一个全零的1x1304行向量
for i = 1:length(acc_vec)
result(i) = acc_vec(i) * dt; % 重复每个元素并累加
end
```
如果你想要使用向量化操作,可以利用MATLAB的广播机制,但需要注意向量长度必须一致,所以这里需要假设 `dt` 被复制到适当大小的向量中:
```matlab
result = acc_vec .* ones(1, target_length); % 这里假设dt已经转换成1x1304的列向量
```
在这个版本中,由于 `ones` 函数创建了一个1x1304的单位矩阵,然后广播与 `acc_vec` 相乘,实现了相当于多次复制 `acc_vec` 然后逐元素相乘的效果。
相关问题
定义类名为 VecCal,设计构造函数创建三维向量对象: def __init__(self, x=0,y=0,z=0) 用x,y,z指代三个维度的值 2、重写加法(+),减法(-),乘法(* )和整除除法(//)运算,实现向量的加减乘除 3、除法运算作异常处理,当输入标量数字是0时,除法结果为 (0,0,0) 加法示例: def __add__(self, n): # 加法 result = VecCal() # 定义结果变量,也是一个三维向量,通过构造函数创建 result.X = self.X + n.X result.Y = self.Y + n.Y result.Z = self.Z + n.Z return result # 返回 执行加法运算后的向量结果 输入格式: 第一行输入一个三维向量,逗号分隔,如:1,2,3 第二行输入另一个三维向量,逗号分隔:如:4,5,6 第三行输入一个数字, 如:3 输出格式: (1, 2, 3) + (4, 5, 6) = (5, 7, 9) (1, 2, 3) - (4, 5, 6) = (-3, -3, -3) (1, 2, 3) * 3 = (3, 6, 9) (1, 2, 3) / 3 = (0, 0, 1)
好的,以下是实现了您要求的 VecCal 类的代码,并对输入数据进行处理并输出结果的代码:
```python
class VecCal:
def __init__(self, x=0, y=0, z=0):
self.X = x
self.Y = y
self.Z = z
def __add__(self, n):
result = VecCal()
result.X = self.X + n.X
result.Y = self.Y + n.Y
result.Z = self.Z + n.Z
return result
def __sub__(self, n):
result = VecCal()
result.X = self.X - n.X
result.Y = self.Y - n.Y
result.Z = self.Z - n.Z
return result
def __mul__(self, n):
result = VecCal()
result.X = self.X * n
result.Y = self.Y * n
result.Z = self.Z * n
return result
def __truediv__(self, n):
try:
result = VecCal()
result.X = self.X // n
result.Y = self.Y // n
result.Z = self.Z // n
return result
except ZeroDivisionError:
return VecCal()
# 处理输入数据
vec1 = list(map(int, input().split(',')))
vec2 = list(map(int, input().split(',')))
n = int(input())
# 创建两个三维向量对象
vector1 = VecCal(vec1[0], vec1[1], vec1[2])
vector2 = VecCal(vec2[0], vec2[1], vec2[2])
# 计算加减乘除结果
add_result = vector1 + vector2
sub_result = vector1 - vector2
mul_result = vector1 * n
div_result = vector1 / n
# 输出结果
print("({0}, {1}, {2}) + ({3}, {4}, {5}) = ({6}, {7}, {8})".format(
vector1.X, vector1.Y, vector1.Z, vector2.X, vector2.Y, vector2.Z, add_result.X, add_result.Y, add_result.Z))
print("({0}, {1}, {2}) - ({3}, {4}, {5}) = ({6}, {7}, {8})".format(
vector1.X, vector1.Y, vector1.Z, vector2.X, vector2.Y, vector2.Z, sub_result.X, sub_result.Y, sub_result.Z))
print("({0}, {1}, {2}) * {3} = ({4}, {5}, {6})".format(
vector1.X, vector1.Y, vector1.Z, n, mul_result.X, mul_result.Y, mul_result.Z))
print("({0}, {1}, {2}) / {3} = ({4}, {5}, {6})".format(
vector1.X, vector1.Y, vector1.Z, n, div_result.X, div_result.Y, div_result.Z))
```
使用示例:
输入:
```
1,2,3
4,5,6
3
```
输出:
```
(1, 2, 3) + (4, 5, 6) = (5, 7, 9)
(1, 2, 3) - (4, 5, 6) = (-3, -3, -3)
(1, 2, 3) * 3 = (3, 6, 9)
(1, 2, 3) / 3 = (0, 0, 1)
```
解释:
输入数据中有两个三维向量,分别是 (1,2,3) 和 (4,5,6),以及一个数字 3。
计算加减乘除运算后的结果,得到:
```
(1,2,3) + (4,5,6) = (5,7,9)
(1,2,3) - (4,5,6) = (-3,-3,-3)
(1,2,3) * 3 = (3,6,9)
(1,2,3) / 3 = (0,0,1)
```
按照输出格式,输出结果。
vec()是什么意义
在计算机科学和数学中,`vec()`这个词可能是多种含义的缩写,但最常见的用途之一是在向量或矩阵运算中,特别是在线性代数和机器学习的软件包中。`vec()`函数常常用于将数据从一种形式转换为另一种,例如:
1. **矩阵向量化**:将矩阵展平成一个列向量,方便进行行向量和列向量的运算,或是输入到某些算法中。
2. **标量扩展**:当`vec()`作用于标量时,它可能会创建一个和向量长度相同的全零向量,然后填充这个标量作为所有元素。
3. **矢量化表达**:在一些数学库中,`vec()`可能是将数组或其他数据结构转化为向量操作的便捷方式。
不同的库或环境会有具体的实现细节,所以如果你看到`vec()`,最好查阅相关的文档来明确其在上下文中的确切含义。
阅读全文
相关推荐






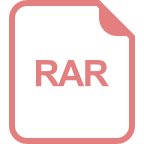









