三元矩阵表的加法运算代码
时间: 2024-10-18 19:15:57 浏览: 23
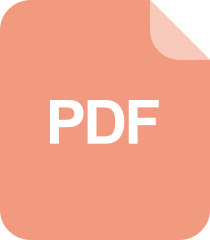
三元组表矩阵的高效运算方法与编程实现
三元矩阵(也称为布尔矩阵或指示矩阵)是一种特殊的二维数组,其元素通常是0(表示False)或1(表示True)。这种矩阵通常用于表示集合之间的关系,如并集、交集等。对于三元矩阵的加法,我们不需要像处理常规数值矩阵那样,因为它们只有两个非零值。简单来说,如果对应位置的元素一个是1,另一个也是1,结果就是1;其他情况保持不变,仍然是0。
下面是一个简单的Python示例,展示了如何对两个三元矩阵进行加法操作:
```python
# 定义两个3x3的三元矩阵
matrix1 = [[0, 1, 1], [1, 0, 1], [1, 1, 0]]
matrix2 = [[1, 1, 0], [0, 1, 1], [1, 0, 1]]
# 对应位置相加
result_matrix = [[matrix1[i][j] + matrix2[i][j] for j in range(len(matrix1[0]))] for i in range(len(matrix1))]
print(result_matrix)
```
这个例子中,`result_matrix`将会存储加法后的结果,其中每个位置的结果取决于原矩阵中相应位置元素的和是否为1。
阅读全文
相关推荐
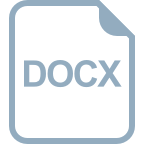
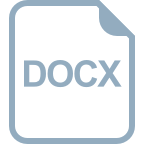
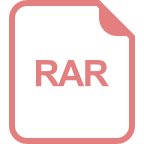
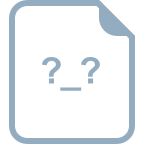
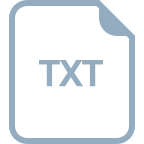
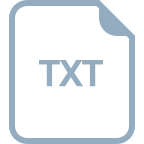
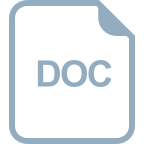
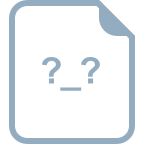
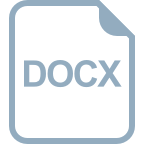
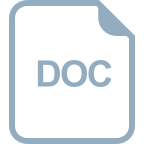
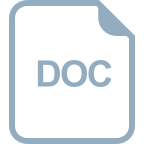





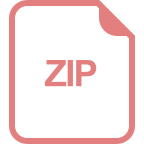