利用dcmtk简单实现c-move 代码
时间: 2023-09-27 18:02:09 浏览: 84
DCMTK是一个用于医学影像处理的开源软件,支持DICOM(Digital Imaging and Communications in Medicine)标准。实现C-MOVE是其中的一个功能,它允许从DICOM服务器上获取医学影像数据。
下面是一个使用DCMTK简单实现C-MOVE的代码示例:
```cpp
#include "dcmtk/dcmnet/assoc.h"
#include "dcmtk/dcmnet/cond.h"
#include "dcmtk/dcmnet/dicom.h"
int main()
{
// 初始化DCMTK库
DcmNetSCU.initializeNetwork();
// 创建一个新的DICOM关联
T_ASC_Parameters* params;
ASC_createAssociationParameters(¶ms);
ASC_setAPTitles(params, "C-MOVE_SCU", "C-MOVE_SCP", NULL);
ASC_setTransportLayerType(params, TL_TCP);
ASC_setAcceptor(params, OFTrue);
// 连接DICOM服务器
const char* serverHostname = "127.0.0.1";
const int serverPort = 11112;
T_ASC_Association* assoc;
ASC_requestAssociation(params, &assoc, serverHostname, serverPort);
if(ASC_associationAccepted(assoc))
{
// 发送C-MOVE请求
DcmDataset* queryDataSet = new DcmDataset();
// 设置查询条件,例如StudyInstanceUID、SeriesInstanceUID等
queryDataSet->putAndInsertString(DCM_QueryRetrieveLevel, "STUDY");
queryDataSet->putAndInsertString(DCM_StudyInstanceUID, "1.2.3.4.5");
T_DIMSE_C_MoveRQ moveRequest;
memset(&moveRequest, 0, sizeof(moveRequest));
moveRequest.MessageID = assoc->nextMsgID++;
strncpy(moveRequest.AffectedSOPClassUID, UID_FINDStudyRootQueryRetrieveInformationModel, sizeof(moveRequest.AffectedSOPClassUID));
OFString moveDest = "C-MOVE_SCP"; // C-MOVE_SCP的AETitle
OFString moveDestHost;
OFString moveDestPort;
OFStandard::strExtractSubstring(moveDest, moveDestHost, 0, OFStandard::strChr(moveDest, ':'));
OFStandard::strExtractSubstring(moveDest, moveDestPort, OFStandard::strChr(moveDest, ':') + 1, OFString_npos);
strncpy(moveRequest.MoveDestination, moveDestHost.c_str(), sizeof(moveRequest.MoveDestination));
moveRequest.MoveDestination[15] = '\0';
moveRequest.Priority = DIMSE_PRIORITY_LOW;
moveRequest.Identifier = queryDataSet;
DIMSE_sendMoveRequest(assoc, &moveRequest, NULL, NULL, NULL);
// 处理C-MOVE响应
T_DIMSE_C_MoveRSP* moveResponse;
while(DIMSE_receiveCommand(assoc, DIMSE_NONBLOCKING, 0) == DIMSE_NORMAL)
{
DIMSE_receiveDataSetInMemory(assoc, DIMSE_NONBLOCKING, NULL, NULL, NULL);
DIMSE_getResponse(assoc, params, &moveResponse);
// 解析响应,可以获取到影像数据
}
delete moveResponse;
delete queryDataSet;
}
else
{
// 关联未被接受
}
// 关闭关联并清理资源
ASC_releaseAssociation(assoc);
ASC_destroyAssociation(&assoc);
// 清理DCMTK库
DcmNetSCU.cleanupNetwork();
return 0;
}
```
以上代码会连接到一个运行在本地的DICOM服务器,并发送一个C-MOVE请求,然后接收服务器返回的C-MOVE响应。在发送请求时,我们需要设置查询的条件,例如StudyInstanceUID、SeriesInstanceUID等来指定要获取的医学影像数据。在响应处理时,可以解析返回的数据集以获取影像数据。
以上是一个很简单的C-MOVE实现示例,实际应用中可能会有更多的细节和错误处理。使用DCMTK库可以方便地实现DICOM相关的功能,包括C-MOVE等。
相关推荐
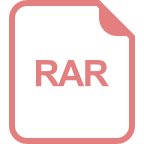
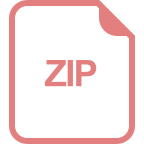
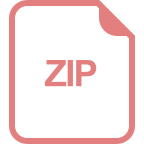














