本关任务: 编程实现英文词频统计: (1)从键盘输入文件名 (2)读取文件中内容 (3)统计文件中英文单词的个数 (4)降序输出单词及个数
时间: 2024-12-20 17:27:48 浏览: 9
在Python中,你可以使用内置的`collections`模块来实现这个任务。以下是一个简单的示例:
```python
import re
from collections import Counter
def word_frequency(input_file):
# (1) 从键盘输入文件名
file_name = input("请输入文件名: ")
try:
with open(file_name, 'r', encoding='utf-8') as file:
# (2) 读取文件内容
content = file.read()
except FileNotFoundError:
print(f"文件 {file_name} 不存在.")
return
# (3) 统计文件中英文单词的个数
words = re.findall(r'\b\w+\b', content.lower()) # 使用正则表达式提取单词并转小写
word_counts = Counter(words)
# (4) 降序输出单词及个数
for word, count in word_counts.most_common():
print(f"{word}: {count}")
# 调用函数开始统计
word_frequency("your_file.txt")
```
在这个脚本中,我们首先让用户输入文件名,然后尝试打开并读取文件内容。接着,使用正则表达式`\b\w+\b`找到所有英文单词,并将它们转换为小写以忽略大小写的差异。利用`Counter`类计算单词频率,最后使用`most_common()`方法按频率降序打印结果。
阅读全文
相关推荐
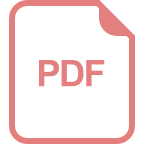
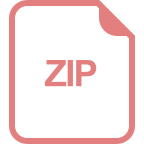
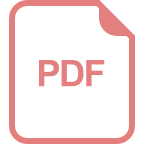


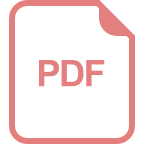
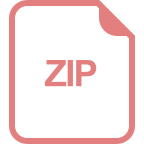
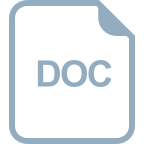
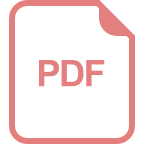
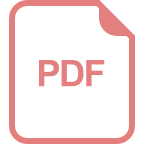
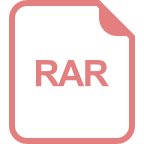
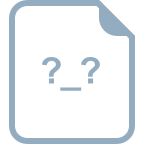
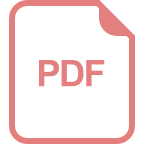
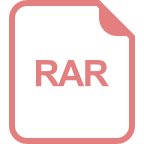
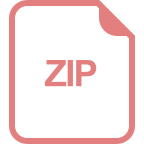
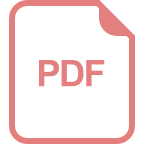