在没有第三方库的支持下用python写一个神经卷积示例
时间: 2024-09-15 08:15:10 浏览: 60
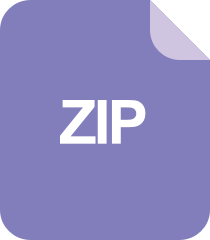
PureNeuralNetwork:从头开始编写的神经网络,没有特殊的第三方库
在Python中编写一个简单的神经网络卷积层示例,通常我们会使用深度学习框架如TensorFlow或PyTorch,因为它们提供了内置的卷积层功能。如果没有第三方库支持,我们可以手动实现一个基于numpy的基础卷积操作。下面是一个基础的二维卷积的例子:
```python
import numpy as np
def convolution(input_data, kernel):
# 假设输入数据是4D数组 (batch_size, height, width, channels)
# 卷积核也是4D数组 (filter_height, filter_width, in_channels, out_channels)
batch_size, in_height, in_width, in_channels = input_data.shape
filter_height, filter_width, _, out_channels = kernel.shape
output = np.zeros((batch_size, in_height - filter_height + 1, in_width - filter_width + 1, out_channels))
for i in range(in_height - filter_height + 1):
for j in range(in_width - filter_width + 1):
patch = input_data[:, i:i+filter_height, j:j+filter_width, :]
output[:, i, j, :] = np.sum(patch * kernel, axis=(0, 1, 2))
return output
# 示例数据
input_data = np.random.rand(1, 5, 5, 3) # 一个batch大小为1、高宽各5的RGB图像
kernel = np.random.rand(3, 3, 3, 1) # 一个3x3的小滤波器,out_channels=1
convolved_output = convolution(input_data, kernel)
```
这只是一个非常基础的版本,实际应用中会包含更复杂的操作,比如步长(strides)、填充(padding)以及批处理归一化等。如果你想深入了解如何在高级框架中使用卷积层,推荐学习TensorFlow或PyTorch的官方文档。
阅读全文
相关推荐
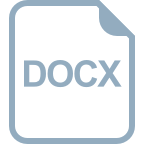
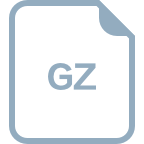
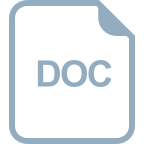


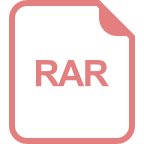
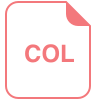
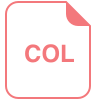
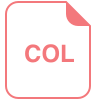
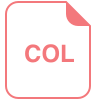
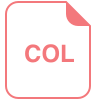
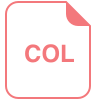
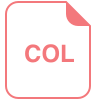
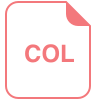
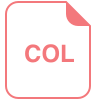

