vba 将ole数据流转换成bmp图片
时间: 2024-09-18 19:19:03 浏览: 70
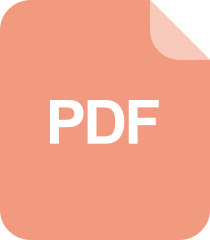
将excel数据转换成dataset示例
在VBA中,将OLE数据流(如嵌入的图像或链接的图形文件)转换为BMP位图格式通常需要两个步骤:首先,你需要从OLEDATA中提取图像数据,然后使用Windows API函数或第三方库(如FreeImage)将其转换为BMP。
1. 提取图像数据:
对于嵌入的图片,可以使用`Shape.Data`或`Shape.OleFormat.Object`来获取图像数据。如果使用的是链接的图片,则可能需要先下载再处理。
```vba
Sub ConvertToBMP()
Dim oleData As Variant
oleData = ActiveSheet.Shapes(1).Data '假设是第一个形状内的图片
'如果图片是链接的,先下载到临时文件
If IsEmpty(oleData) Then
oleData = DownloadLinkedImage() '自定义的下载函数
End If
If TypeOf oleData Is Byte() Then
'oleData现在是一个字节数组,包含了图片数据
Else
Debug.Print "Invalid data type"
Exit Sub
End If
End Sub
```
2. 转换为BMP:
接下来,你可以使用`CreateFromStream`函数配合`FreeImage`库将字节数组转换为BMP。这里是一个简单的示例,假设已经引入了`FreeImage`库:
```vba
Function ConvertBytesToBMP(byteArray() As Byte) As Bitmap
Dim bi As FreeImage.BITMAPINFO
Dim dibSection As Long
Dim hBitmap As Long
bi.biSize = Len(bi)
bi.biWidth = FreeImageGetWidth(byteArray, 0)
bi.biHeight = -FreeImageGetHeight(byteArray, 0) '注意高度是负数表示顶部开始坐标
bi.biPlanes = 1
bi.biBitCount = 24 'BMP是24位色彩
bi.biCompression = FreeImage.FICF_RGB
bi.biSizeImage = bi.biWidth * bi.biHeight * bi.biBitCount / 8
bi.biXPelsPerMeter = 0
bi.biYPelsPerMeter = 0
bi.biClrUsed = 0
bi.biClrImportant = 0
ReDim buffer(LBound(byteArray) To UBound(byteArray)) As Integer '临时数组用于存储像素值
For i = LBound(byteArray) To UBound(byteArray)
buffer(i) = (byteArray(i) And &HFF) << 16 | (byteArray(i + 1) And &HFF) << 8 | (byteArray(i + 2) And &HFF)
Next i
Set dibSection = GlobalPtr.CreateDIBSection(hDC, ByVal ByRef bi, FreeImage.DIB_PAL_COLORS, ByVal 0, ByVal 0, FreeImage.DIB_RGB_COLORS)
Call FreeImage.LoadMemory(FreeImage.FI_RGBA, dibSection, Len(buffer))
hBitmap = FreeImage.GetDIBHandle(dibSection)
Set ConvertBytesToBMP = CreateObject("Bitmap")
ConvertBytesToBMP.Picture = LoadPicture(longPtr(hBitmap), "PNG")
FreeImage.Unload(hBitmap)
End Function
```
这里的`hDC`是画布设备上下文,一般情况下不需要手动创建,如果你有特定需求,可以查阅文档找到正确的初始化方式。
阅读全文
相关推荐
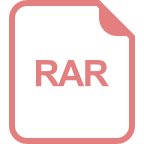
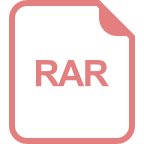


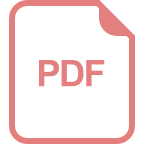
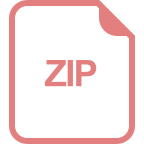
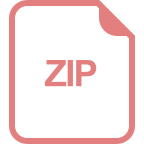
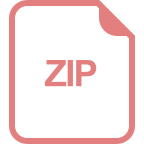
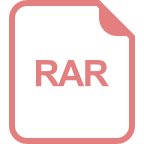
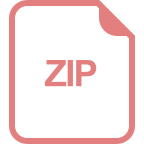
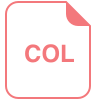




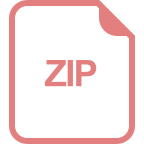