python录像邮件发送
时间: 2023-10-29 09:54:07 浏览: 118
为了实现Python的录像邮件发送功能,您可以按照以下步骤进行操作:
1. 导入所需的库:您需要导入`time`、`cv2`、`smtplib`、`MIMEImage`、`MIMEText`和`MIMEMultipart`库。
2. 调用摄像头并保存图像:使用`cv2.VideoCapture`函数调用您的摄像头。在循环中,使用`cap.read()`读取摄像头的每一帧图像,然后使用`cv2.imshow()`显示图像。通过按下空格键,使用`cv2.imwrite()`将当前帧保存为图像文件。
3. 构造邮件内容:使用`MIMEImage`和`MIMEText`类构造邮件的内容。将保存的图像作为附件添加到邮件中,并设置邮件的文本内容。
4. 发送邮件:使用`smtplib.SMTP()`函数连接到SMTP服务器,并使用`login()`函数登录到您的邮箱。然后,使用`sendmail()`函数将邮件发送到指定的邮箱地址。最后,使用`quit()`函数断开与SMTP服务器的连接。
下面是一个示例代码,可以帮助您实现这个功能:
```python
import time
import cv2
from email.mime.image import MIMEImage
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
import smtplib
def send_email(msg):
smtp = smtplib.SMTP()
smtp.connect('smtp.163.com', port=25) # 请根据您的邮箱设置选择合适的SMTP服务器和端口号
smtp.login('sender@example.com', 'password') # 请替换为您的发件人邮箱和密码
smtp.sendmail('sender@example.com', 'receiver@example.com', msg.as_string()) # 请替换为您的发件人邮箱和收件人邮箱
smtp.quit()
def capture_and_send():
cap = cv2.VideoCapture(0) # 请根据您的摄像头索引进行调整
while True:
ret, frame = cap.read()
cv2.imshow('Camera', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
elif cv2.waitKey(1) & 0xFF == ord('s'):
cv2.imwrite('image.jpg', frame)
break
cap.release()
cv2.destroyAllWindows()
msg = MIMEMultipart()
msg.attach(MIMEText('Please see the attached image.'))
with open('image.jpg', 'rb') as f:
img = MIMEImage(f.read())
img.add_header('Content-Disposition', 'attachment', filename='image.jpg')
msg.attach(img)
send_email(msg)
if __name__ == '__main__':
capture_and_send()
```
请注意,在上述代码中,您需要将以下内容替换为您自己的信息:
- SMTP服务器和端口号
- 发件人邮箱和密码
- 收件人邮箱
- 摄像头索引(如果有多个摄像头)
您可以根据需要对代码进行调整和改进,以适应您的具体要求。
阅读全文
相关推荐
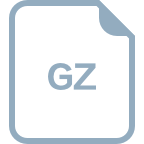
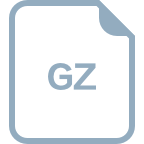










