C++中除了使用字符串流之外还有其他方法获取整型变量的数字长度吗?
时间: 2024-09-06 10:08:15 浏览: 62
在C++中,除了使用字符串流以外,我们还可以使用以下方法来获取整型变量的数字长度:
1. 使用`std::to_string`函数转换整型变量为字符串,然后获取字符串的长度。这种方法实际上也依赖于字符串流的内部实现,但提供了一个更直接的接口。
```cpp
#include <iostream>
#include <string>
int main() {
int number = 12345;
std::string number_str = std::to_string(number);
std::cout << "整型变量的数字长度为: " << number_str.length() << std::endl;
return 0;
}
```
2. 使用`std::stringstream`进行操作,这是一种类似于字符串流的方法,不过它使用的是C++标准库中的流。
```cpp
#include <iostream>
#include <sstream>
#include <string>
int main() {
int number = 12345;
std::stringstream ss;
ss << number;
std::cout << "整型变量的数字长度为: " << ss.str().length() << std::endl;
return 0;
}
```
3. 自己编写循环函数来计算数字的位数。这种方法不依赖于任何标准库提供的流或字符串处理功能,而是通过数学计算的方式确定数字长度。
```cpp
#include <iostream>
int main() {
int number = 12345;
int length = 0;
int temp = number;
if (temp == 0) {
std::cout << "整型变量的数字长度为: 1" << std::endl;
return 0;
}
while (temp > 0) {
length++;
temp /= 10;
}
std::cout << "整型变量的数字长度为: " << length << std::endl;
return 0;
}
```
阅读全文
相关推荐
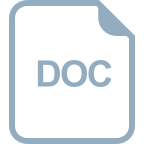
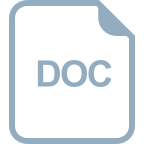
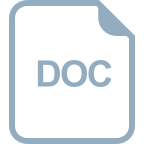

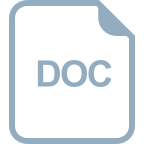
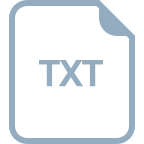
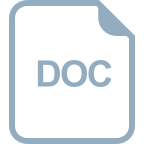
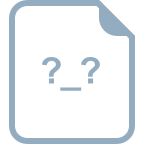
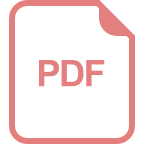
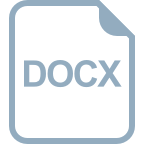
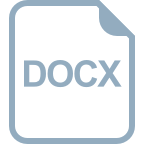
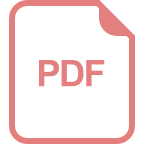
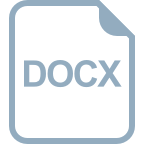
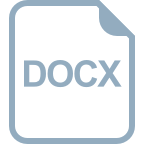
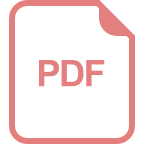
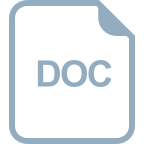
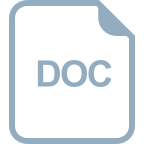
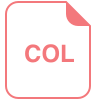
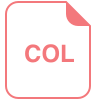