java,通过文件URL,将文件放入压缩包,并将压缩包通过浏览器下载到,请帮我写一个通用的方法,入参是文件url及自定义压缩包名称
时间: 2024-10-10 08:16:52 浏览: 33
在Java中,我们可以使用`java.util.zip`包下的`ZipOutputStream`类来创建压缩包,然后利用`java.net.URL`获取文件内容并通过HTTP服务器提供下载。以下是一个通用的方法示例:
```java
import java.io.*;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class FileCompressor {
public static String downloadFileFromUrl(String urlString, String zipFileName) throws IOException {
URL url = new URL(urlString);
try (InputStream in = url.openStream();
ZipOutputStream out = new ZipOutputStream(new BufferedOutputStream(responseOutputStream(zipFileName))) ) {
byte[] buffer = new byte[4096];
int bytesRead;
// 获取文件名
String fileName = extractFileNameFromUrl(url);
ZipEntry entry = new ZipEntry(fileName);
out.putNextEntry(entry);
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
out.closeEntry();
}
return zipFileName;
}
private static OutputStream responseOutputStream(String zipFileName) {
try {
Path filePath = Paths.get(zipFileName);
if (!Files.exists(filePath)) {
Files.createDirectories(filePath.getParent());
}
return new FileOutputStream(zipFileName);
} catch (IOException e) {
throw new RuntimeException("Failed to create output stream for the compressed file", e);
}
}
private static String extractFileNameFromUrl(URL url) {
String path = url.getPath();
int lastIndexOfQuestionMark = path.lastIndexOf('?');
if (lastIndexOfQuestionMark >= 0) {
path = path.substring(0, lastIndexOfQuestionMark);
}
int lastSlash = path.lastIndexOf('/');
if (lastSlash > 0) {
return path.substring(lastSlash + 1);
} else {
return path;
}
}
}
```
使用这个方法,你可以像这样调用:
```java
try {
String url = "http://example.com/path/to/file.txt";
String zipName = "compressed_file.zip";
String downloadedZipPath = FileCompressor.downloadFileFromUrl(url, zipName);
System.out.println("Downloaded and compressed file saved as: " + downloadedZipPath);
} catch (IOException e) {
e.printStackTrace();
}
```
阅读全文
相关推荐









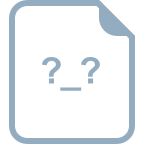




