邮件已下载好,不需要联网
时间: 2024-10-20 19:09:44 浏览: 14
如果你已经下载好了邮件并且不需要联网来查看它们,你可以将邮件存储在本地文件夹中,然后编写一个程序来读取这些文件。这里有一个简单的Python脚本,它假设邮件是以特定格式(例如,Mbox或.eml格式)存储在目录下的:
```python
import os
import email
from email.header import decode_header
def read_local_email(directory):
# 获取目录下所有的邮件文件
email_files = [f for f in os.listdir(directory) if f.endswith('.eml') or f.endswith('.mbox')]
messages = []
for file_name in email_files:
with open(os.path.join(directory, file_name), 'rb') as f:
message = email.message_from_file(f)
messages.append(process_email(message))
return messages
def process_email(message):
# 解析邮件信息
subject, encoding = decode_header(message['Subject'])[0]
if isinstance(subject, bytes):
subject = subject.decode(encoding)
sender = email.utils.parseaddr(message['From'])[1]
body = ''
if message.is_multipart():
for part in message.walk():
if part.get_content_type() == 'text/plain':
body += part.get_payload().decode('utf-8')
else:
body = message.get_payload().decode('utf-8')
return {
'subject': subject,
'sender': sender,
'body': body
}
# 使用函数读取本地邮件
local_directory = '/path/to/your/local/email/folder'
emails = read_local_email(local_directory)
for email in emails:
print(f"Subject: {email['subject']}, Sender: {email['sender']} - Body: {email['body']}")
```
在这个例子中,你需要替换`'/path/to/your/local/email/folder'`为你的实际邮件存储路径。这个脚本会遍历文件夹中的每个邮件文件,将其解析成结构化的字典,包含邮件的主题、发件人和正文。
阅读全文
相关推荐
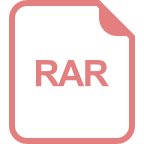
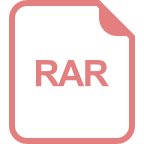
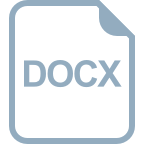
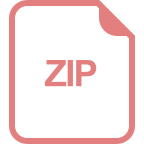
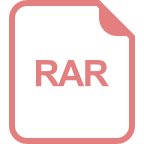
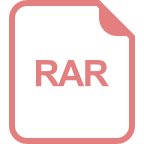
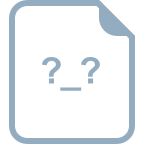
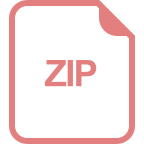
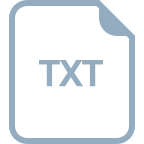
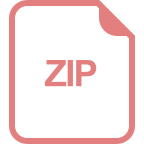
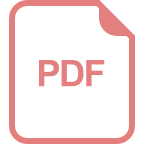
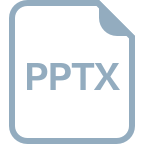
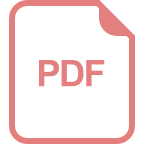
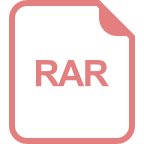
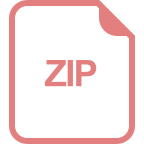
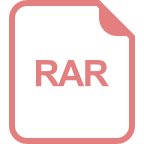
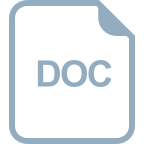
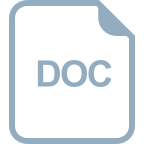