Object.keys()
时间: 2024-08-24 09:00:44 浏览: 32
`Object.keys()` 是JavaScript内置的一个方法,它接收一个对象作为参数,并返回该对象的所有自身可枚举属性的名称组成的数组。这个数组包含了对象的键,而不是值。例如,如果你有一个对象:
```javascript
const person = {
name: "Alice",
age: 30,
city: "New York"
};
```
使用 `Object.keys(person)`,你会得到一个包含 ["name", "age", "city"] 的数组,表示 `person` 对象的所有属性名。
这个方法常用于遍历对象、检查某个对象是否有特定的属性,或者获取对象属性名列表,以便进一步操作或处理这些属性。例如:
```javascript
// 遍历并打印对象的所有属性名
for (let key of Object.keys(person)) {
console.log(key);
}
// 检查对象是否存在某个属性
if (Object.keys(person).includes("age")) {
console.log("Age is a property");
}
```
相关问题
Object.keys
Object.keys() 是一个 JavaScript 中的内置函数,用于返回一个对象自身的所有可枚举属性的属性名组成的数组。可枚举属性是指那些通过 for...in 循环和 Object.keys() 方法能够获取到的属性,而不包括那些不可枚举的属性。该函数的语法为:
```
Object.keys(obj)
```
其中,obj 表示要返回属性名数组的对象。该函数返回的数组中的元素顺序和使用 for...in 循环遍历该对象时返回的属性名顺序一致。例如:
```
const obj = { a: 1, b: 2, c: 3 };
console.log(Object.keys(obj)); // 输出 ["a", "b", "c"]
```
注意,Object.keys() 只返回对象自身的属性名,不包括从原型链继承的属性。如果要获取所有可枚举属性,包括继承的属性,可以使用 for...in 循环。
object.keys
Object.keys() is a built-in JavaScript method that returns an array of the object's property names. It takes an object as an argument and returns an array of the property names of that object. The returned array contains only the property names that are directly owned by the object and not inherited from its prototype chain. The order of the property names in the array is not guaranteed and may vary depending on the JavaScript engine.
For example, consider the following object:
```
const person = {
firstName: 'John',
lastName: 'Doe',
age: 30,
email: 'john.doe@example.com'
};
```
We can use Object.keys() to get an array of the property names of the person object:
```
const keys = Object.keys(person);
console.log(keys); // Output: ['firstName', 'lastName', 'age', 'email']
```
We can then use this array to loop through the object's properties, or access a specific property using bracket notation:
```
keys.forEach(key => {
console.log(person[key]); // Output: 'John', 'Doe', 30, 'john.doe@example.com'
});
console.log(person[keys[0]]); // Output: 'John'
```
相关推荐
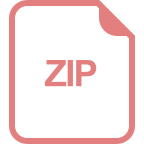
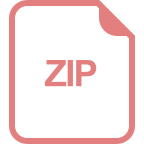
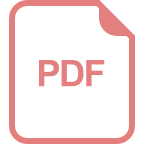










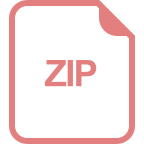
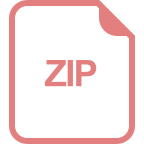
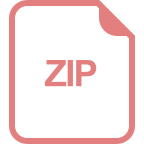