用c++代码实现处理机调度算法
时间: 2024-10-18 20:12:24 浏览: 35
在C++中实现处理器调度算法,例如经典的抢占式优先级级聵(Preemptive Priority Scheduling),可以使用互斥量、条件变量等同步机制。这里给出一个简单的例子,展示了如何使用`std::priority_queue`结合抢占式调度的基本思路:
```cpp
#include <iostream>
#include <queue>
#include <mutex>
#include <condition_variable>
// 定义任务结构体,包含ID、优先级和是否运行中的标志
struct Task {
int id;
int priority;
bool running;
};
// 使用C++11的原子操作管理任务的状态
class Scheduler {
private:
std::priority_queue<Task, std::vector<Task>, std::greater<int>> tasks; // 优先级队列
std::mutex mtx; // 互斥锁
std::condition_variable cond; // 条件变量
public:
void add_task(Task task) {
std::lock_guard<std::mutex> lock(mtx);
tasks.push(task);
if (!task.running && tasks.top().running) { // 如果有新任务且当前任务未运行
notify_start();
}
}
void run() {
while (true) {
std::unique_lock<std::mutex> lock(mtx);
cond.wait(lock, [this] { return !tasks.empty() && tasks.top().running; }); // 等待新任务或非空队列且任务在运行
Task current_task = tasks.top(); // 获取当前最高优先级任务
tasks.pop();
// 这里假设run函数是一个模拟执行的任务操作
run_task(current_task.id);
current_task.running = false; // 任务结束标记
if (!tasks.empty()) {
notify_start(); // 如果还有其他任务,唤醒下一个
}
}
}
void start_task(int id) {
Task task{id, 0, true}; // 初始化任务为运行状态
{
std::lock_guard<std::mutex> lock(mtx);
tasks.push(task);
}
cond.notify_one(); // 唤醒运行函数
}
void run_task(int id) {
std::cout << "Running task: " << id << std::endl;
// 模拟任务实际执行逻辑
// ...
}
private:
void notify_start() {
cond.notify_one(); // 唤醒run函数检查是否有新任务
}
};
int main() {
Scheduler scheduler;
scheduler.add_task({1, 5, false});
scheduler.add_task({2, 3, false});
scheduler.start_task(1); // 强制启动第一个任务
scheduler.run(); // 开始调度
// ... 更多任务添加和start_task操作...
return 0;
}
```
这个例子中,`Scheduler`类负责管理任务队列,并通过`add_task`添加任务,`start_task`用于开始一个新的任务。`run`函数不断从队列中取出最高优先级的任务并运行,直到队列为空。注意这只是一个简化版本,实际应用中可能会更复杂,包括错误处理和更多调度策略。
阅读全文
相关推荐
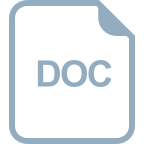
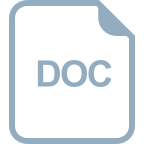
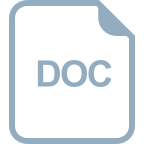

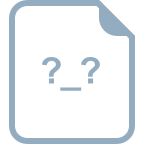
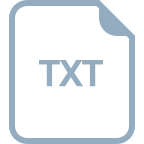
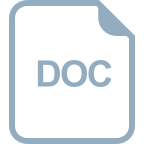
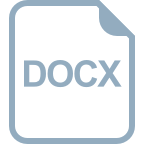
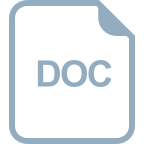

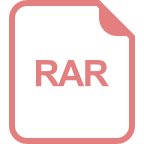
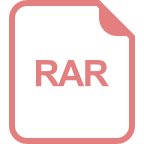
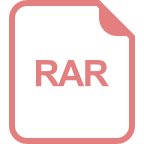
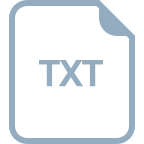
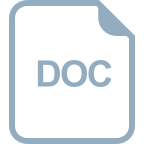

