c#中创建array<array>类型数组
时间: 2023-12-09 08:06:31 浏览: 35
在 C# 中,您可以使用多维数组或者嵌套的数组来创建 array<array> 类型的数组。以下是两种常见的方法:
1. 多维数组:
```csharp
T[,] myArray = new T[M, N];
```
这里的 T 是数组中元素的类型,M 是第一维度的大小,N 是第二维度的大小。请将 T 替换为实际的数据类型。
2. 嵌套的数组:
```csharp
T[][] myArray = new T[M][];
for (int i = 0; i < M; i++)
{
myArray[i] = new T[N];
}
```
这里的 T 是数组中元素的类型,M 是外部数组的大小,N 是内部数组的大小。使用循环为每个内部数组分配内存。
请注意,上述代码中的 `<T>` 部分应该被替换为您实际希望在数组中存储的数据类型。
相关问题
C# 如何创建Array对象
在C#中,可以通过以下方式来创建Array对象:
1. 使用Array.CreateInstance方法来创建数组,语法如下:
```csharp
Array arrayName = Array.CreateInstance(type, length);
```
其中,type是数组类型,length是数组长度。
例如,创建一个包含5个整数类型的数组:
```csharp
int[] intArray = (int[])Array.CreateInstance(typeof(int), 5);
```
2. 使用数组字面量(array initializer)来创建数组,语法如下:
```csharp
type[] arrayName = {value1, value2, ..., valueN};
```
例如,创建一个包含3个字符串类型的数组:
```csharp
string[] strArray = {"apple", "banana", "orange"};
```
3. 直接创建数组对象,语法如下:
```csharp
type[] arrayName = new type[length];
```
例如,创建一个包含4个双精度类型的数组:
```csharp
double[] dblArray = new double[4];
```
无论哪种方法,都可以创建一个指定类型和长度的数组对象。
array类型数组变量可以接受不同类型变量作为元素吗
在 C# 中,Array 是一个强类型数组,也就是说,一个 Array 类型的变量只能存储相同类型的元素。如果尝试将不同类型的变量赋值给一个 Array 类型的变量,编译器就会报错。
例如,下面的代码会导致编译错误:
```csharp
// 定义一个包含不同类型元素的数组
object[] array = { 1, "hello", true };
```
因为上面的数组包含了 int、string 和 bool 类型的元素,这些元素的类型不同,因此编译器会报错。
如果想要存储不同类型的元素,可以使用 C# 中的泛型集合类,例如 List。List 可以存储不同类型的元素,而且还提供了很多实用的方法,可以更方便地操作集合。例如,下面的代码可以成功编译:
```csharp
// 定义一个包含不同类型元素的 List
List<object> list = new List<object> { 1, "hello", true };
```
相关推荐
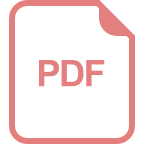
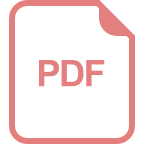
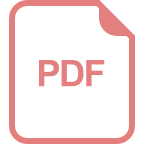












