handleOk () { const that = this this.$refs.modalForm.validate((valid) => { if (valid) { let obj if (!this.formData.module_id) { // 新增 obj = api.functionAdd(this.params) } else { // 单行编辑 obj = this.type !== '2' ? api.functionEdit(this.params) : api.functionCopy(this.params, this.params.module_id) } obj .then((res) => { if (res.success) { that.$message.success(res.message) that.$emit('ok', this.type) } else { that.$message.error(res.message) } }) .finally(() => { that.handleCancel() }) } }) },优化以上代码
时间: 2024-02-14 11:29:11 浏览: 128
你可以优化以上代码的部分。首先,你可以使用箭头函数的隐式返回来简化代码。在`then`和`finally`回调中,可以直接返回Promise对象,而不是将其赋值给`obj`变量。像这样:
```javascript
handleOk() {
this.$refs.modalForm.validate((valid) => {
if (valid) {
let obj;
if (!this.formData.module_id) {
// 新增
obj = api.functionAdd(this.params);
} else {
// 单行编辑
obj = this.type !== '2' ? api.functionEdit(this.params) : api.functionCopy(this.params, this.params.module_id);
}
return obj
.then((res) => {
if (res.success) {
this.$message.success(res.message);
this.$emit('ok', this.type);
} else {
this.$message.error(res.message);
}
})
.finally(() => {
this.handleCancel();
});
}
});
}
```
此外,你可以使用箭头函数来绑定`that`变量。这样就不需要在函数内部创建`that`变量并赋值给`this`。只需直接使用`this`来访问组件实例。像这样:
```javascript
handleOk() {
this.$refs.modalForm.validate((valid) => {
if (valid) {
let obj;
if (!this.formData.module_id) {
// 新增
obj = api.functionAdd(this.params);
} else {
// 单行编辑
obj = this.type !== '2' ? api.functionEdit(this.params) : api.functionCopy(this.params, this.params.module_id);
}
return obj
.then((res) => {
if (res.success) {
this.$message.success(res.message);
this.$emit('ok', this.type);
} else {
this.$message.error(res.message);
}
})
.finally(() => {
this.handleCancel();
});
}
});
}
```
最后,确保你在代码中使用合适的缩进和格式化,以提高可读性。
阅读全文
相关推荐








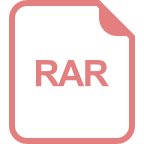








