在个人中心界面设置一个打卡按钮,每天只能打卡一次,直到另一天才能继续打卡,并将数据传到数据库,数据库有id,打卡用户id,打卡时间,第几天打卡的界面,写出springboot+vue代码
时间: 2024-12-17 08:24:27 浏览: 4
在Spring Boot项目中,我们可以使用Spring Data JPA结合Vue.js来实现这样的功能。首先,我们需要创建实体(Entity)、服务(Service)、Repository以及Vue页面。
**1. Entity (实体类) - UserCheckin.java**
```java
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class UserCheckin {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private Long userId; // 用户ID
private LocalDateTime checkinTime; // 打卡时间
private int day; // 当前打卡的天数
// getters and setters...
}
```
**2. Service (服务类) - CheckinService.java**
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.Date;
import java.time.LocalDateTime;
@Service
public class CheckinService {
@Autowired
private UserRepository userRepository;
public void dailyCheckin(Long userId) {
if (!isUserCheckedInToday(userId)) {
UserCheckin userCheckin = new UserCheckin();
userCheckin.setUserId(userId);
userCheckin.setDay(new Date().getDay() + 1); // 获取当前日期是一周中的第几天
userCheckin.setCheckinTime(LocalDateTime.now());
userRepository.save(userCheckin);
} else {
throw new IllegalStateException("今日已打卡");
}
}
private boolean isUserCheckedInToday(Long userId) {
return userRepository.existsByUserIdAndDay(userId, new Date().getDay() + 1);
}
}
```
**3. Repository (仓库接口) - UserRepository.java**
```java
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends JpaRepository<UserCheckin, Long> {
boolean existsByUserIdAndDay(Long userId, int day);
}
```
**4. Vue 页面 (组件或路由文件)** - 在`/templates/daily-checkin.vue` 或者相应的模板文件中
```html
<template>
<button @click="checkin">今日打卡</button>
</template>
<script>
export default {
methods: {
checkin() {
axios.post('/api/checkin', { userId: this.userId }) // 假设userId存储在vuex里
.then(() => {
// 更新数据库状态(这里需要前端与后端交互)
// 如果有错误处理逻辑,可以添加异常捕获
})
.catch(error => console.error(error));
},
},
};
</script>
```
**
阅读全文
相关推荐
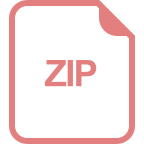
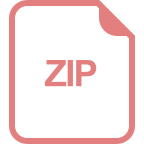
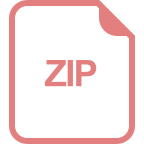
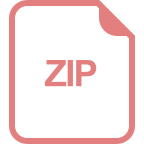
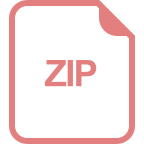
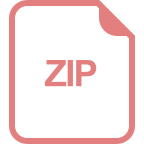
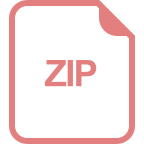
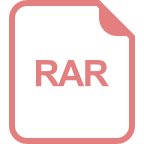
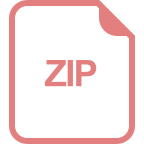
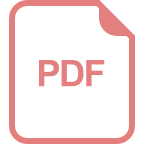
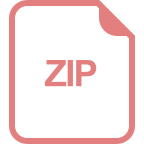