帮我写一个社团管理系统,用C语言和数据结构
时间: 2024-09-19 15:14:41 浏览: 51
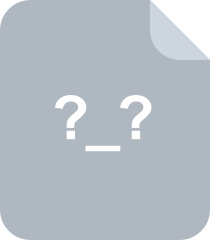
社团管理系统C语言控制台应用程序
创建一个社团管理系统,我们可以采用C语言和一些基础的数据结构,如数组、链表或结构体来存储用户信息和社团信息。这里是一个简单的示例,我们将使用链表作为主数据结构:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义社团结构体
typedef struct Club {
char name[50];
int members;
struct Club* next; // 链接指针
} Club;
// 用户结构体
typedef struct User {
char username[50];
char password[50]; // 这里仅作示例,实际应用需考虑安全措施
Club* affiliated_clubs; // 关联到的社团
} User;
// 功能函数
Club* createClub(char* clubName) {
Club* newClub = (Club*)malloc(sizeof(Club));
strcpy(newClub->name, clubName);
newClub->members = 0;
newClub->next = NULL;
return newClub;
}
void addUserToClub(User* user, Club* club) {
Club** current = &user->affiliated_clubs;
while (*current != NULL) {
current = &(*current)->next;
}
*current = club;
++club->members;
}
int main() {
// 初始化示例
Club* club1 = createClub("计算机科学爱好者");
// 添加用户到俱乐部
User user1;
strcpy(user1.username, "Alice");
strcpy(user1.password, "password1");
addUserToClub(&user1, club1);
// 打印社团成员
printf("Club '%s' has %d member(s):", club1->name, club1->members);
Club* temp = club1;
while (temp != NULL) {
printf("%s ", temp->name); // 假设每个社团只有一个名称
temp = temp->next;
}
return 0;
}
```
阅读全文
相关推荐
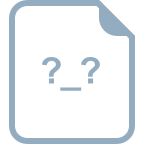
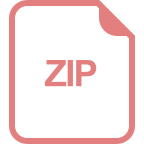
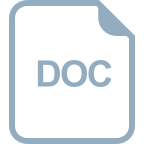
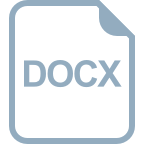
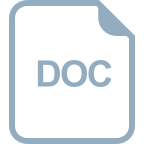
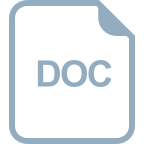
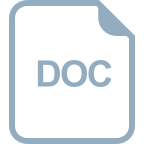
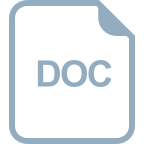
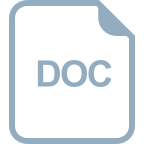
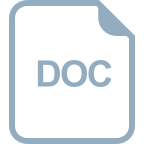
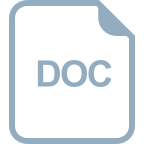
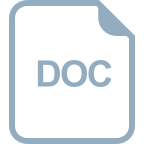
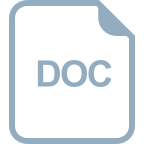




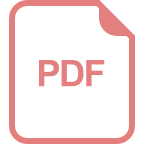
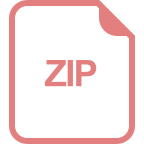