Python百例练习之第十八
时间: 2023-11-19 18:49:19 浏览: 87
Python百例练习之第十八例是一个回文数判断的实例。下面是代码的解释:
引用的代码片段是一个判断回文数的函数。首先,它定义了一个名为palindrome的函数,该函数接受一个字符串作为参数。然后,函数通过将字符串进行反转,并将反转后的字符串与原字符串进行比较来判断是否是回文数。如果两个字符串相等,则打印出该字符串是一个回文数,否则打印出该字符串不是一个回文数。
接下来,引用中的代码片段使用了一个for循环,循环次数为3次。在每次循环中,它通过input函数接收用户输入的一个正整数,并调用palindrome函数来判断该正整数是否是回文数。
相关问题
python简单练习
在Python中,简单的练习可以帮助初学者巩固基础并熟悉语言特性。这里有一些常见的Python练习:
1. **Hello World**:编写一个小程序打印 "Hello, World!",这是学习任何编程语言的第一个步骤。
```python
print("Hello, World!")
```
2. **变量和数据类型**:尝试创建、赋值和操作不同类型的变量,比如整数(int)、浮点数(float)、字符串(str)等。
```python
num = 5
float_num = 3.14
text = "Python is fun"
```
3. **条件语句**:理解并使用 `if`、`else` 和 `elif` 来做出决策。
```python
age = 20
if age >= 18:
print("You are an adult")
else:
print("You are a minor")
```
4. **循环结构**:了解 `for` 循环遍历列表,以及 `while` 循环的基本用法。
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
i = 0
while i < 5:
print(i)
i += 1
```
5. **函数**:编写和调用简单的函数,封装常用的操作。
```python
def greet(name):
return f"Hello, {name}!"
greeting = greet("Alice")
print(greeting)
```
6. **列表操作**:学习如何添加、删除元素,以及切片和索引。
```python
numbers = [1, 2, 3, 4, 5]
numbers.append(6)
print(numbers[0])
numbers.remove(3)
print(numbers[::2]) # 每两个取一个
```
python if else练习题
好的,以下是 Python if else 练习题:
1. 编写一个程序,判断一个数是否为偶数。
```python
num = int(input("请输入一个整数:"))
if num % 2 == 0:
print(num, "是偶数")
else:
print(num, "不是偶数")
```
2. 编写一个程序,判断一个年份是否为闰年。
```python
year = int(input("请输入一个年份:"))
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
print(year, "是闰年")
else:
print(year, "不是闰年")
```
3. 编写一个程序,输入三个数,输出其中最大的数。
```python
a = float(input("请输入第一个数:"))
b = float(input("请输入第二个数:"))
c = float(input("请输入第三个数:"))
if a >= b and a >= c:
print(a, "是最大的数")
elif b >= a and b >= c:
print(b, "是最大的数")
else:
print(c, "是最大的数")
```
4. 编写一个程序,输入三个数,输出其中最小的数。
```python
a = float(input("请输入第一个数:"))
b = float(input("请输入第二个数:"))
c = float(input("请输入第三个数:"))
if a <= b and a <= c:
print(a, "是最小的数")
elif b <= a and b <= c:
print(b, "是最小的数")
else:
print(c, "是最小的数")
```
5. 编写一个程序,输入一个字符,判断它是大写字母、小写字母还是数字。
```python
ch = input("请输入一个字符:")
if ch.isupper():
print(ch, "是大写字母")
elif ch.islower():
print(ch, "是小写字母")
elif ch.isdigit():
print(ch, "是数字")
else:
print(ch, "不是大写字母、小写字母或数字")
```
6. 编写一个程序,输入一个年龄,判断它是否为儿童(0-12岁)、青少年(13-18岁)或成年人(19岁及以上)。
```python
age = int(input("请输入一个年龄:"))
if age < 0:
print("输入有误")
elif age <= 12:
print("儿童")
elif age <= 18:
print("青少年")
else:
print("成年人")
```
7. 编写一个程序,输入一个月份,输出该月份的天数。
```python
month = int(input("请输入一个月份:"))
if month in [1, 3, 5, 7, 8, 10, 12]:
print(month, "月有31天")
elif month in [4, 6, 9, 11]:
print(month, "月有30天")
elif month == 2:
year = int(input("请输入一个年份:"))
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
print(month, "月有29天")
else:
print(month, "月有28天")
else:
print("输入有误")
```
阅读全文
相关推荐
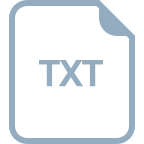
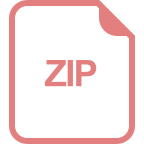
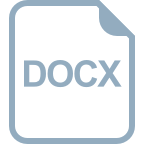
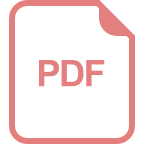
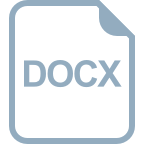
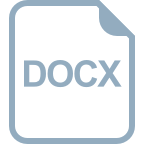
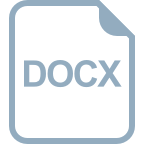
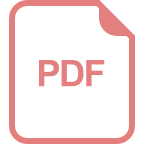
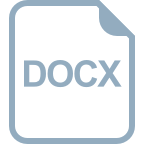
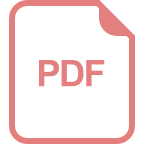
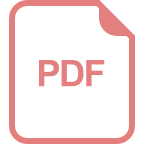
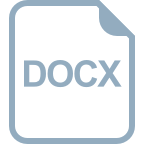
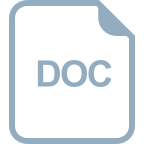
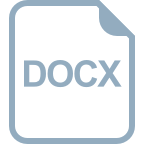