用C语言编写集合的交并差
时间: 2024-09-10 10:26:11 浏览: 26
在C语言中,由于其基础性,没有内置的数据结构库直接提供集合操作如交集、并集和差集的功能。然而,你可以手动创建数据结构,比如使用数组或链表来模拟这些操作。以下是一个简单的示例,我们可以通过哈希表(这里使用`struct Set`表示)来实现:
```c
#include <stdbool.h>
#include <stdlib.h>
// 创建一个哈希表作为集合的基本数据结构
typedef struct Set {
size_t size;
void **hash_table;
} Set;
// 初始化集合
Set *set_create() {
Set *s = (Set*) malloc(sizeof(Set));
s->size = 0;
s->hash_table = (void**) calloc(100, sizeof(void*)); // 假设初始容量为100
return s;
}
// 插入元素到集合
void set_insert(Set *s, void *elem) {
size_t index = hash_function(elem); // 使用哈希函数计算元素位置
if (!s->hash_table[index]) {
s->hash_table[index] = elem;
s->size++;
}
}
// 判断元素是否在集合中
bool set_contains(Set *s, void *elem) {
size_t index = hash_function(elem);
return s->hash_table[index] == elem;
}
// 计算两个集合的交集
Set *set_intersection(Set *a, Set *b) {
Set *result = set_create();
for (size_t i = 0; i < a->size; i++) {
if (set_contains(b, a->hash_table[i])) {
set_insert(result, a->hash_table[i]);
}
}
return result;
}
// 计算两个集合的并集
Set *set_union(Set *a, Set *b) {
Set *result = set_create();
for (size_t i = 0; i < a->size || i < b->size; i++) {
if (i < a->size && !set_contains(b, a->hash_table[i])) {
set_insert(result, a->hash_table[i]);
} else if (i < b->size && !set_contains(a, b->hash_table[i])) {
set_insert(result, b->hash_table[i]);
}
}
return result;
}
// 计算两个集合的差集
Set *set_difference(Set *a, Set *b) {
Set *result = set_create();
for (size_t i = 0; i < a->size; i++) {
if (!set_contains(b, a->hash_table[i])) {
set_insert(result, a->hash_table[i]);
}
}
return result;
}
// 清理集合资源
void set_destroy(Set *s) {
free(s->hash_table);
free(s);
}
// 哈希函数示例,实际应用中需自行实现
size_t hash_function(void *elem) {
// 这里仅作演示,实际需要复杂算法保证散列均匀
return (size_t) elem;
}
```
相关推荐
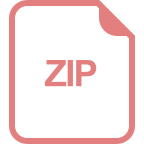
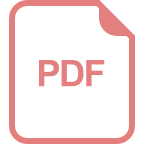














