计算机包含内存(ram)、cpu等硬件设备,根据图片所示“产品等级结构-产品族”示意图,使用抽象工厂模式实现计算机设备创建过程并绘制相应的类
时间: 2023-10-17 10:02:42 浏览: 374
抽象工厂模式是一种创建对象的设计模式,它通过提供一个接口来创建一系列相关或相互依赖的对象,而无需指定具体的类。
在进行计算机设备的创建过程中,可以使用抽象工厂模式来实现。首先,在抽象工厂模式中需要定义一个抽象工厂接口,用于创建计算机设备的各个部件。该接口中可以定义创建内存和CPU的方法。
```java
interface ComputerFactory {
Memory createMemory();
CPU createCPU();
}
```
然后,根据具体的产品族,实现具体的工厂类和产品类。例如,创建Intel产品族的工厂:
```java
class IntelComputerFactory implements ComputerFactory {
public Memory createMemory() {
return new IntelMemory();
}
public CPU createCPU() {
return new IntelCPU();
}
}
```
创建AMD产品族的工厂:
```java
class AMDComputerFactory implements ComputerFactory {
public Memory createMemory() {
return new AMDMemory();
}
public CPU createCPU() {
return new AMDCPU();
}
}
```
同时,需要定义每个具体产品的类。例如,创建内存和CPU的抽象类:
```java
abstract class Memory {
}
abstract class CPU {
}
```
再具体实现Intel和AMD的内存和CPU类:
```java
class IntelMemory extends Memory {
// 具体实现省略
}
class IntelCPU extends CPU {
// 具体实现省略
}
class AMDMemory extends Memory {
// 具体实现省略
}
class AMDCPU extends CPU {
// 具体实现省略
}
```
最后,在客户端中使用抽象工厂来创建计算机设备:
```java
class Client {
public static void main(String[] args) {
ComputerFactory factory = new IntelComputerFactory();
Memory memory = factory.createMemory();
CPU cpu = factory.createCPU();
// 根据需要进行后续操作
}
}
```
以上就是使用抽象工厂模式实现计算机设备创建过程的示例,根据图片所示的产品等级结构,可以根据具体的产品族来实现对应的工厂类和产品类。
阅读全文
相关推荐




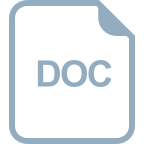







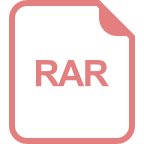

