野狗优化算法python代码
时间: 2024-06-11 18:02:45 浏览: 30
野狗优化算法是一种基于种群的全局优化算法,其灵感来源于野狗捕食时的行为。这种算法的主要特点是通过不断的迭代和自适应调整来找到最优解。下面是野狗优化算法的Python代码示例:
```python
import numpy as np
class WOA:
def __init__(self, func, dim, max_iter=100, lb=-100, ub=100, population=10):
self.func = func
self.dim = dim
self.max_iter = max_iter
self.lb = lb
self.ub = ub
self.population = population
def init_population(self):
return np.random.uniform(low=self.lb, high=self.ub, size=(self.population, self.dim))
def get_fitness(self, population):
fitness = np.zeros(self.population)
for i in range(self.population):
fitness[i] = self.func(population[i])
return fitness
def update_position(self, population, leader_pos):
a = 2 - 2 * np.linspace(0, 1, self.max_iter) ** 2
r1 = np.random.rand(self.population, self.dim)
r2 = np.random.rand(self.population, self.dim)
A = 2 * a * r1 - population)
new_population = leader_pos - A * D
return new_population
def run(self):
population = self.init_population()
fitness = self.get_fitness(population)
best_index = np.argmin(fitness)
best_fitness = fitness[best_index]
best_position = population[best_index]
for i in range(self.max_iter):
a = 2 - 2 * (i / (self.max_iter - 1)) ** 2
A = 2 * a * np.random.rand(self.dim) - a
C = 2 * np.random.rand(self.dim)
b = 1
l = (a - 1) * np.random.rand() + 1
p = np.random.rand()
if p < 0.5:
if np.abs(A) < 1:
new_population = self.update_position(population, best_position)
else:
rand_leader_index = np.random.randint(0, self.population)
rand_leader_pos = population[rand_leader_index]
new_population = self.update_position(population, rand_leader_pos)
else:
distance_to_leader = np.abs(C * best_position - population)
new_population = distance_to_leader * np.exp(b * l) * np.cos(2 * np.pi * l) + best_position
new_population[new_population < self.lb] = self.lb
new_population[new_population > self.ub] = self.ub
new_fitness = self.get_fitness(new_population)
new_best_index = np.argmin(new_fitness)
if new_fitness[new_best_index] < best_fitness:
best_fitness = new_fitness[new_best_index]
best_position = new_population[new_best_index]
population = new_population
fitness = new_fitness
return best_position, best_fitness
```
以上是一个简单的野狗优化算法的Python实现,需要提供目标函数、维度、最大迭代次数、上下界和种群大小等参数。在`run()`方法中进行算法的迭代,并返回最优解及其对应的函数值。
相关推荐
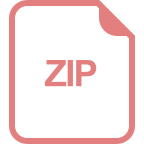














