thinkphp 请求第三方接口
时间: 2025-01-01 12:27:13 浏览: 25
### 如何在 ThinkPHP 中调用第三方 API 接口
#### 准备工作
为了成功调用第三方 API,在项目中需先安装 `guzzlehttp/guzzle` 库来简化 HTTP 请求操作。通过 Composer 安装此库:
```bash
composer require guzzlehttp/guzzle
```
#### 创建服务类用于发起请求
创建一个新的 PHP 类文件,比如命名为 `ApiService.php` 放置在应用的服务目录下 (`application/service`)。
```php
<?php
namespace app\service;
use GuzzleHttp\Client;
use think\Exception;
class ApiService {
protected $client;
private $baseUrl = "https://api.example.com"; // 替换成实际API的基础URL
public function __construct() {
try{
$this->client = new Client(['base_uri' => $this->baseUrl]);
} catch (Exception $e){
throw new Exception('Failed to create client:' . $e->getMessage());
}
}
/**
* 发起GET请求获取数据.
*/
public function get($endpoint, array $params = []) {
try {
$response = $this->client->request('GET', $endpoint, ['query' => $params]);
return json_decode((string)$response->getBody(), true);
} catch (\GuzzleHttp\Exception\RequestException $e) {
throw new \RuntimeException('Error during GET request: ' . $e->getMessage());
}
}
}
```
上述代码展示了如何构建一个简单的 API 服务类[^1]。
#### 控制器中的使用方法
接下来是在控制器里实例化并使用这个服务类来进行 API 调用的例子:
```php
<?php
namespace app\controller;
use app\service\ApiService;
use think\Controller;
class ApiController extends Controller {
public function fetchDataFromThirdParty(){
$apiService = new ApiService();
try {
$data = $apiService->get('/some-endpoint'); // 假设这是你要访问的端点
if ($data !== null && isset($data['success']) && !$data['success']){
echo "Request failed with message:" . $data['message'];
}else{
var_dump($data); // 输出返回的数据结构供调试查看
}
}catch(\Exception $ex){
echo "An error occurred while fetching data from third party api:".$ex->getMessage();
}
}
}
```
这段代码说明了怎样在一个控制器动作内初始化 `ApiService` 并执行具体的 API 方法[^4]。
阅读全文
相关推荐













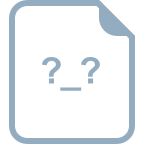




