python rotate
时间: 2023-08-25 09:18:07 浏览: 63
旋转一个 Python 列表可以使用切片操作来实现。你可以使用以下代码来进行列表的旋转:
```python
def rotate_list(lst, k):
n = len(lst)
k = k % n
return lst[k:] + lst[:k]
# 示例用法
my_list = [1, 2, 3, 4, 5]
rotation_amount = 2
rotated_list = rotate_list(my_list, rotation_amount)
print(rotated_list)
```
在上面的代码中,`rotate_list` 函数接受一个列表 `lst` 和一个旋转的数量 `k`。它首先计算出列表的长度 `n`,然后对 `k` 进行取模以确保旋转量在列表长度范围内。最后,它将切片 `[k:]`(从第 `k` 个元素到列表末尾)和切片 `[:k]`(从列表开头到第 `k` 个元素)连接起来,得到旋转后的列表。
在示例中,原始列表 `[1, 2, 3, 4, 5]` 被旋转了 2 个位置,得到了旋转后的列表 `[3, 4, 5, 1, 2]`。你可以根据实际需要修改 `my_list` 和 `rotation_amount` 的值来尝试不同的旋转效果。
相关问题
python rotate函数
`rotate` 函数可以用来旋转列表或字符串中的元素。它接受两个参数:要旋转的对象和旋转的步数。正数表示向右旋转,负数表示向左旋转。
以下是一个示例代码,演示了如何使用 `rotate` 函数来旋转列表:
```python
def rotate(lst, steps):
length = len(lst)
steps = steps % length # 处理 steps 大于列表长度的情况
if steps == 0:
return lst
rotated_lst = lst[-steps:] + lst[:-steps]
return rotated_lst
# 示例用法
my_list = [1, 2, 3, 4, 5]
rotated_list = rotate(my_list, 2) # 向右旋转2步
print(rotated_list) # 输出: [4, 5, 1, 2, 3]
```
类似地,你也可以使用这个函数来旋转字符串。例如:
```python
def rotate(string, steps):
length = len(string)
steps = steps % length
if steps == 0:
return string
rotated_string = string[-steps:] + string[:-steps]
return rotated_string
# 示例用法
my_string = "Hello, World!"
rotated_string = rotate(my_string, -3) # 向左旋转3步
print(rotated_string) # 输出: "lo, World!Hel"
```
希望这可以帮助到你!如果你有任何其他问题,请随时提问。
python rotate函数的使用
Python的rotate函数用于旋转列表中的元素。它接受一个整数参数,表示要旋转的元素数量。如果该参数是正数,列表将向右旋转;如果该参数是负数,列表将向左旋转。以下是一个示例:
```python
lst = [1, 2, 3, 4, 5]
lst.rotate(2) # 向右旋转2个元素
print(lst) # 输出:[4, 5, 1, 2, 3]
lst.rotate(-1) # 向左旋转1个元素
print(lst) # 输出:[5, 1, 2, 3, 4]
```
需要注意的是,rotate函数是在原列表上进行修改,而不是返回一个新列表。因此,如果需要保留原列表,可以使用copy函数创建一个副本。例如:
```python
lst = [1, 2, 3, 4, 5]
lst_copy = lst.copy() # 创建一个副本
lst.rotate(2) # 向右旋转2个元素
print(lst) # 输出:[4, 5, 1, 2, 3]
print(lst_copy) # 输出:[1, 2, 3, 4, 5]
```
相关推荐
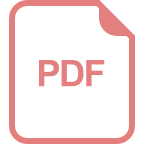
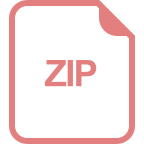
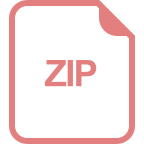












