jupyternotebok 中怎么解压文件夹
时间: 2024-10-08 19:08:22 浏览: 12
在Jupyter Notebook中解压文件夹通常涉及到使用Python的内置模块如`shutil`或第三方库`zipfile`。这里是一个简单的步骤说明:
1. 首先,你需要导入所需的库,例如`shutil`:
```python
import shutil
```
2. 使用`shutil`的`unpack_archive()`函数来解压缩文件。假设你想解压名为`my_folder.zip`的文件到当前工作目录下,你可以这样做:
```python
shutil.unpack_archive('my_folder.zip', '.') # '.' 表示当前目录作为提取目标
```
或者如果你知道具体的目标文件夹路径,可以替换为那个路径。
3. 如果需要解压的是ZIP格式,还可以使用`zipfile`库:
```python
from zipfile import ZipFile
with ZipFile('my_folder.zip', 'r') as zip_ref:
zip_ref.extractall('.') # 提取所有内容到当前目录
```
记得替换实际的文件名和路径。解压完成后,文件夹将出现在指定的位置。
相关问题
jupyternotebok采用BP神经网络模型将鸢尾花数据二分类。
首先,加载鸢尾花数据集并进行预处理:
```python
import numpy as np
from sklearn import datasets
iris = datasets.load_iris()
X = iris.data
y = iris.target
# 将标签转换为0和1
y[y != 0] = 1
# 标准化特征值
X = (X - np.mean(X, axis=0)) / np.std(X, axis=0)
```
然后,我们定义BP神经网络模型。在这个例子中,我们使用一个具有两个隐藏层的神经网络,每个隐藏层有10个神经元。我们使用sigmoid作为激活函数,二元交叉熵作为损失函数,批量梯度下降作为优化算法。
```python
class BPNeuralNetwork:
def __init__(self, n_input, n_hidden1, n_hidden2, n_output):
self.n_input = n_input
self.n_hidden1 = n_hidden1
self.n_hidden2 = n_hidden2
self.n_output = n_output
# 初始化权重和偏置
self.weights1 = np.random.randn(self.n_input, self.n_hidden1)
self.bias1 = np.zeros((1, self.n_hidden1))
self.weights2 = np.random.randn(self.n_hidden1, self.n_hidden2)
self.bias2 = np.zeros((1, self.n_hidden2))
self.weights3 = np.random.randn(self.n_hidden2, self.n_output)
self.bias3 = np.zeros((1, self.n_output))
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(self, x):
return x * (1 - x)
def forward(self, X):
self.z1 = np.dot(X, self.weights1) + self.bias1
self.a1 = self.sigmoid(self.z1)
self.z2 = np.dot(self.a1, self.weights2) + self.bias2
self.a2 = self.sigmoid(self.z2)
self.z3 = np.dot(self.a2, self.weights3) + self.bias3
self.y_hat = self.sigmoid(self.z3)
return self.y_hat
def backward(self, X, y, y_hat, learning_rate):
# 计算输出层误差
self.output_error = y - y_hat
self.output_delta = self.output_error * self.sigmoid_derivative(y_hat)
# 计算隐藏层2误差
self.hidden2_error = np.dot(self.output_delta, self.weights3.T)
self.hidden2_delta = self.hidden2_error * self.sigmoid_derivative(self.a2)
# 计算隐藏层1误差
self.hidden1_error = np.dot(self.hidden2_delta, self.weights2.T)
self.hidden1_delta = self.hidden1_error * self.sigmoid_derivative(self.a1)
# 更新权重和偏置
self.weights1 += learning_rate * np.dot(X.T, self.hidden1_delta)
self.bias1 += learning_rate * np.sum(self.hidden1_delta, axis=0, keepdims=True)
self.weights2 += learning_rate * np.dot(self.a1.T, self.hidden2_delta)
self.bias2 += learning_rate * np.sum(self.hidden2_delta, axis=0, keepdims=True)
self.weights3 += learning_rate * np.dot(self.a2.T, self.output_delta)
self.bias3 += learning_rate * np.sum(self.output_delta, axis=0, keepdims=True)
def train(self, X, y, learning_rate=0.1, n_epochs=1000):
for i in range(n_epochs):
y_hat = self.forward(X)
self.backward(X, y, y_hat, learning_rate)
def predict(self, X):
y_hat = self.forward(X)
predictions = np.round(y_hat)
return predictions
```
接下来,我们将数据集分为训练集和测试集,并训练模型。
```python
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
bpnn = BPNeuralNetwork(n_input=4, n_hidden1=10, n_hidden2=10, n_output=1)
bpnn.train(X_train, y_train, learning_rate=0.1, n_epochs=1000)
```
最后,我们使用测试集评估模型。
```python
from sklearn.metrics import accuracy_score
predictions = bpnn.predict(X_test)
accuracy = accuracy_score(y_test, predictions)
print("Accuracy:", accuracy)
```
完整代码如下:
```python
import numpy as np
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
class BPNeuralNetwork:
def __init__(self, n_input, n_hidden1, n_hidden2, n_output):
self.n_input = n_input
self.n_hidden1 = n_hidden1
self.n_hidden2 = n_hidden2
self.n_output = n_output
# 初始化权重和偏置
self.weights1 = np.random.randn(self.n_input, self.n_hidden1)
self.bias1 = np.zeros((1, self.n_hidden1))
self.weights2 = np.random.randn(self.n_hidden1, self.n_hidden2)
self.bias2 = np.zeros((1, self.n_hidden2))
self.weights3 = np.random.randn(self.n_hidden2, self.n_output)
self.bias3 = np.zeros((1, self.n_output))
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(self, x):
return x * (1 - x)
def forward(self, X):
self.z1 = np.dot(X, self.weights1) + self.bias1
self.a1 = self.sigmoid(self.z1)
self.z2 = np.dot(self.a1, self.weights2) + self.bias2
self.a2 = self.sigmoid(self.z2)
self.z3 = np.dot(self.a2, self.weights3) + self.bias3
self.y_hat = self.sigmoid(self.z3)
return self.y_hat
def backward(self, X, y, y_hat, learning_rate):
# 计算输出层误差
self.output_error = y - y_hat
self.output_delta = self.output_error * self.sigmoid_derivative(y_hat)
# 计算隐藏层2误差
self.hidden2_error = np.dot(self.output_delta, self.weights3.T)
self.hidden2_delta = self.hidden2_error * self.sigmoid_derivative(self.a2)
# 计算隐藏层1误差
self.hidden1_error = np.dot(self.hidden2_delta, self.weights2.T)
self.hidden1_delta = self.hidden1_error * self.sigmoid_derivative(self.a1)
# 更新权重和偏置
self.weights1 += learning_rate * np.dot(X.T, self.hidden1_delta)
self.bias1 += learning_rate * np.sum(self.hidden1_delta, axis=0, keepdims=True)
self.weights2 += learning_rate * np.dot(self.a1.T, self.hidden2_delta)
self.bias2 += learning_rate * np.sum(self.hidden2_delta, axis=0, keepdims=True)
self.weights3 += learning_rate * np.dot(self.a2.T, self.output_delta)
self.bias3 += learning_rate * np.sum(self.output_delta, axis=0, keepdims=True)
def train(self, X, y, learning_rate=0.1, n_epochs=1000):
for i in range(n_epochs):
y_hat = self.forward(X)
self.backward(X, y, y_hat, learning_rate)
def predict(self, X):
y_hat = self.forward(X)
predictions = np.round(y_hat)
return predictions
iris = datasets.load_iris()
X = iris.data
y = iris.target
# 将标签转换为0和1
y[y != 0] = 1
# 标准化特征值
X = (X - np.mean(X, axis=0)) / np.std(X, axis=0)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
bpnn = BPNeuralNetwork(n_input=4, n_hidden1=10, n_hidden2=10, n_output=1)
bpnn.train(X_train, y_train, learning_rate=0.1, n_epochs=1000)
predictions = bpnn.predict(X_test)
accuracy = accuracy_score(y_test, predictions)
print("Accuracy:", accuracy)
```
注:这只是一个简单的示例,实际应用中需要进行更多的数据预处理和模型调优。
阅读全文
相关推荐
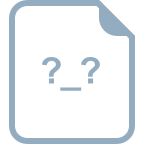
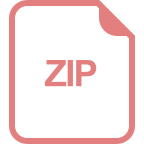
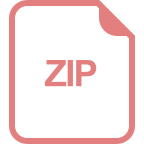
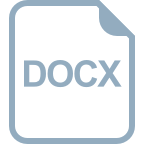
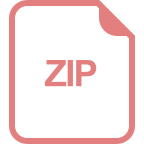
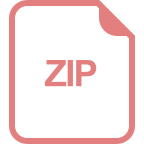
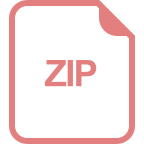
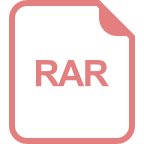
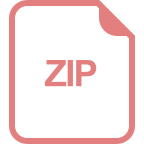
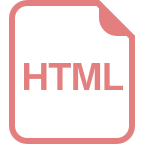
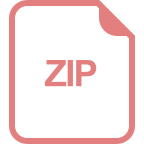