如何用C++编程语言实现一个显示爱心图案的程序?
时间: 2024-11-15 08:25:36 浏览: 44
在C++中,我们可以使用循环结构和控制字符来创建简单的爱心图案。下面是一个基础的示例,它使用星(*)来表示爱心:
```cpp
#include <iostream>
void printHeart(int size) {
for (int i = 0; i < size; ++i) {
for (int j = 0; j <= size - i; ++j) {
std::cout << "*";
}
std::cout << "\n"; // 换行
}
for (int i = size - 1; i >= 0; --i) {
for (int j = 0; j < size - i; ++j) {
std::cout << "*";
}
std::cout << "\n";
}
}
int main() {
int heartSize = 5; // 可以调整爱心的大小
printHeart(heartSize);
return 0;
}
```
在这个程序中,我们首先打印出上半部分的心形,然后印下半部分的心形,通过嵌套循环控制每个*的位置。如果你想得到更复杂的爱心形状(如空心的),你可以稍微修改内部循环条件。
相关问题
如何在C++编程中实现动态绘制爱心图案的代码?
在C++中,动态绘制爱心图案通常涉及到窗口系统API如Windows API或Qt等库,因为标准C++库并不直接支持图形输出。以下是使用Windows GDI+(Graphics Device Interface)的一个简单示例,它在屏幕上绘制爱心:
```cpp
#include <windows.h>
#include <gdiplus.h>
using namespace Gdiplus;
void draw_heart(HDC hdc) {
SolidBrush brush(Color::White);
Graphics graphics(hdc);
// 上半部分心形
for (int y = 0; y <= 4; y++) {
for (int x = -y; x <= 4 + y; x++) {
if (x * x + y * y <= 20) {
graphics.FillEllipse(&brush, x * 5, y * 5, 5, 5);
}
}
graphics.DrawLine(&brush, 0, 5 * (4 - y), 8, 5 * (4 - y));
}
// 下半部分心形
for (int y = 1; y <= 4; y++) {
for (int x = -y + 1; x <= 4 + y; x++) {
if (x * x + (y - 1) * (y - 1) <= 20) {
graphics.FillEllipse(&brush, x * 5, (y - 1) * 5, 5, 5);
}
}
graphics.DrawLine(&brush, 0, (5 * (4 - y) - 1), 8, (5 * (4 - y) - 1));
}
}
int main() {
try {
HINSTANCE hInstance = GetModuleHandle(NULL);
HDC hdcScreen = GetDC(NULL);
HRGN windowRgn = CreateRectRgn(0, 0, GetSystemMetrics(SM_CXSCREEN), GetSystemMetrics(SM_CYSCREEN));
SetWindowRgn(hwndConsole, windowRgn, TRUE);
// 获取控制台的设备上下文
HCONSOLE console = GetStdHandle(STD_OUTPUT_HANDLE);
HDC hdcConsole = GetConsoleWindowDC(console);
HGDIOBJ oldPen = SelectObject(hdcConsole, GetStockObject(BLACK_PEN));
// 开始绘图
draw_heart(hdcConsole);
// 清理资源
DeleteObject(oldPen);
ReleaseDC(console, hdcConsole);
ReleaseDC(hwndConsole, hdcScreen);
DeleteObject(windowRgn);
GdiplusShutdown(gdiplusToken);
return 0;
} catch(...) {
return 1;
}
}
```
注意,这需要在命令行环境下运行,并且GDI+不是跨平台的,如果要在其他平台上做类似操作,你可能需要选择不同的图形库或工具。
如何用C++编程语言编写一个输出爱心形状的代码?
在C++中,你可以使用嵌套循环来创建一个爱心形状的ASCII艺术输出。下面是一个简单的例子:
```cpp
#include <iostream>
using namespace std;
void printHeart() {
int size;
cout << "请输入爱心的大小 (行数): ";
cin >> size;
for(int i = 0; i <= size; ++i) {
// 上半部分
for(int j = 0; j <= i; ++j) {
cout << "*";
}
cout << endl;
// 下半部分:中间一行空
if(i > 0) {
cout << " ";
}
// 下半部分
for(int k = 0; k < 2 * i - 1; ++k) {
cout << "*";
}
cout << endl;
}
}
int main() {
printHeart();
return 0;
}
```
运行这个程序,它会提示用户输入爱心的大小,然后打印出相应大小的心形图案。
阅读全文
相关推荐
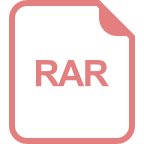
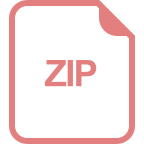
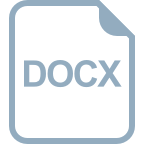
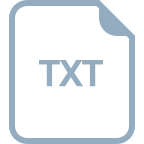
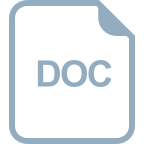
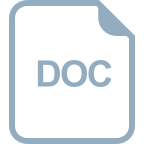
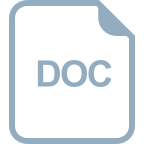
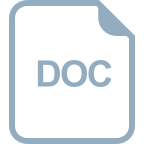
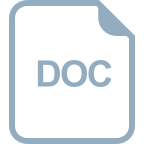
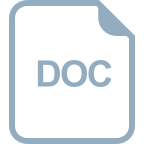







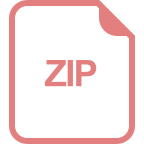