c++单链表头插法和尾插法分别实现插入算法。
时间: 2024-09-27 21:02:23 浏览: 12
在 C++ 中,单链表是一种常见的数据结构,头插法(Push at the front)和尾插法(Push at the end)是两种常用的节点插入操作。
1. **头插法(Insert at beginning)**:
```cpp
struct Node {
int data;
Node* next;
};
void insertAtBeginning(Node*& head, int value) {
// 创建新节点
Node* newNode = new Node();
newNode->data = value;
newNode->next = head; // 将新节点的next指向前一个节点
// 更新头节点指向新节点
head = newNode;
}
```
2. **尾插法(Insert at end)**:
```cpp
void insertAtEnd(Node*& head, int value) {
// 如果链表为空
if (head == nullptr) {
head = new Node();
head->data = value;
head->next = nullptr; // 初始化新链表只有一个节点,其next指针设为nullptr
} else { // 链表非空
Node* current = head;
while (current->next != nullptr) {
current = current->next; // 找到最后一个元素
}
// 在最后一个节点之后插入
current->next = new Node();
current->next->data = value;
current->next->next = nullptr; // 新节点的next指向nullptr,表示这是列表的结尾
}
}
```
相关推荐
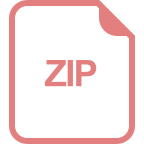
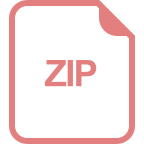
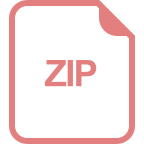














