使用Servlet实现小写字母转大写。提示:创建一个HTML页面用于接收需要转换的字符串,并提交到一个Servlet;创建一个Servlet实现字符串的接收和转换,并将转换后的数据显示到客户端。
时间: 2024-06-12 08:09:05 浏览: 25
使用Servlet实现小写字母转大写的步骤如下:
1. 创建一个HTML页面,包含一个表单和一个文本框,用于接收需要转换的字符串。在表单中设置提交按钮,将表单提交到一个Servlet。
2. 创建一个Servlet,实现字符串的接收和转换。在Servlet的doPost方法中,获取表单提交的字符串参数,并将其转换为大写。
3. 将转换后的数据显示到客户端。可以使用response.getWriter()方法获取输出流,然后使用println方法将转换后的数据输出到客户端。
示例代码如下:
HTML页面(index.html):
```html
<!DOCTYPE html>
<html>
<head>
<title>转换小写字母为大写</title>
</head>
<body>
<form action="ConvertServlet" method="POST">
<input type="text" name="inputString" placeholder="输入需要转换的字符串">
<input type="submit" value="转换">
</form>
</body>
</html>
```
Servlet(ConvertServlet.java):
```java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ConvertServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取表单提交的字符串参数
String inputString = request.getParameter("inputString");
// 将字符串转换为大写
String outputString = inputString.toUpperCase();
// 设置响应类型和编码
response.setContentType("text/html");
response.setCharacterEncoding("UTF-8");
// 输出转换后的数据到客户端
response.getWriter().println("转换后的字符串为:" + outputString);
}
}
```
请注意,为了使Servlet能够正常工作,需要将HTML页面和Servlet代码放置在Web应用程序的正确目录结构下,并配置web.xml文件。
相关推荐
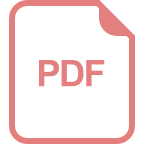
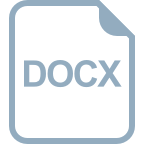
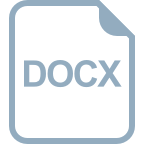














