private boolean checkFireStrength(int maxEntryValue, int maxEntrySize, LinkedList<FireDetectionResult> copyList) { double[] avgStrength = new double[maxEntryValue]; List<Double>[] strengthArray = new ArrayList[maxEntryValue]; for(int i = 0; i < maxEntryValue; i++) { strengthArray[i] = new ArrayList<>(); } for (FireDetectionResult result : copyList) { int currentValue = result.fireAreaList.size(); if (currentValue == maxEntryValue) { for(int i = 0; i < maxEntryValue; i++) { FireArea fire = result.fireAreaList.get(i); avgStrength[i] += fire.strength; strengthArray[i].add(fire.strength); } } } for (int i = 0 ; i < maxEntryValue; i++) { avgStrength[i] /= maxEntrySize; strengthArray[i].sort(Comparator.comparingDouble(o -> o)); if (checkAvgStrength(avgStrength[i]) || checkMaxStrength(strengthArray[i]) || checkMinStrength(strengthArray[i])) { return true; } } return false; }
时间: 2024-02-14 13:30:30 浏览: 22
这段代码的作用是检查火灾的强度是否符合一定的条件。具体实现如下:
- 创建一个长度为 `maxEntryValue` 的 `double` 数组 `avgStrength`,用于保存每个火灾区域的平均强度。
- 创建一个长度为 `maxEntryValue` 的 `List<Double>` 数组 `strengthArray`,用于保存每个火灾区域的强度列表。
- 遍历 `copyList` 中的每个 `FireDetectionResult` 对象。
- 对于每个 `FireDetectionResult` 对象,获取其 `fireAreaList` 的大小,并将其赋值给 `currentValue`。
- 如果 `currentValue` 等于 `maxEntryValue`,表示该结果具有最大条目数,需要进行处理:
- 遍历 `maxEntryValue` 次,对于每个索引 `i`:
- 获取当前结果的第 `i` 个火灾区域,并将其强度加到对应的 `avgStrength[i]` 中。
- 将当前火灾区域的强度添加到对应的 `strengthArray[i]` 中。
- 遍历 `avgStrength` 和 `strengthArray` 中的每个元素:
- 计算每个火灾区域的平均强度,并将结果保存在对应的 `avgStrength[i]` 中。
- 对每个火灾区域的强度列表进行排序。
- 如果满足以下任一条件,则返回 `true`:
- 通过调用 `checkAvgStrength` 方法检查平均强度是否符合要求。
- 通过调用 `checkMaxStrength` 方法检查最大强度是否符合要求。
- 通过调用 `checkMinStrength` 方法检查最小强度是否符合要求。
- 如果没有满足条件的情况,则返回 `false`。
总结起来,该方法根据火灾区域的条目数,计算每个区域的平均强度,并检查这些强度是否满足特定条件。如果满足条件,则返回 `true`,否则返回 `false`。
相关推荐
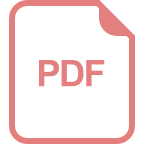
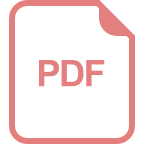















