c语言怎么加载dll
时间: 2024-09-12 22:16:13 浏览: 42
在C语言中,加载动态链接库(DLL)通常通过Windows API函数LoadLibrary和GetProcAddress来完成,如果你是在Linux或Unix-like系统上,可以使用dlopen、dlsym等函数。以下是基本步骤:
**Windows (使用WinAPI):**
```c
#include <windows.h>
HMODULE hModule;
hModule = LoadLibrary("path_to_your_dll.dll");
if (hModule == NULL) {
// 处理加载失败
printf("Failed to load DLL\n");
} else {
FARPROC funcAddress = GetProcAddress(hModule, "function_name"); // 替换为实际函数名
if (funcAddress != NULL) {
// 现在你可以使用funcAddress调用DLL中的函数了
void* result = (*funcAddress)();
// 使用结果
} else {
printf("Failed to get function address\n");
}
FreeLibrary(hModule); // 释放DLL句柄
}
```
**Linux (使用glibc):**
```c
#include <dlfcn.h>
void* handle = dlopen("path_to_your_library.so", RTLD_LAZY | RTLD_GLOBAL);
if (!handle) {
perror("Error loading library");
} else {
void* func = dlsym(handle, "function_name");
if (func) {
// 调用函数
int result = (*static_cast<int (*)(void)>(func))();
} else {
fprintf(stderr, "Function not found\n");
}
dlclose(handle); // 关闭库
}
```
请注意,你需要替换"路径_to_your_dll.dll"或"path_to_your_library.so"为实际的DLL或共享库文件路径,并将"function_name"替换为你想要调用的具体函数名。
阅读全文
相关推荐
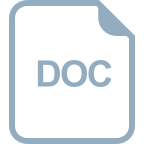
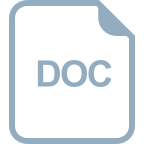
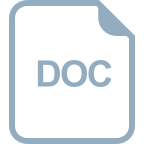
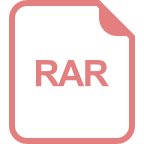
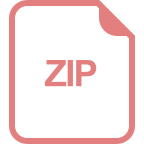
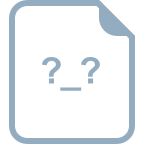
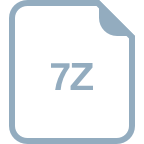
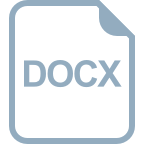
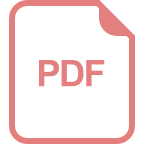
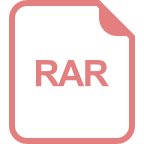
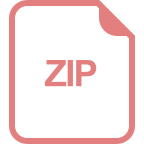
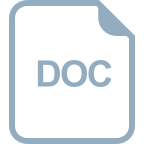
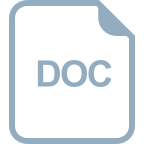




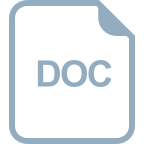
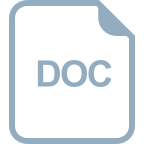
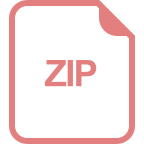