请给出完整Python代码,能够通过用户自定义的数据集进行训练识别手写阿拉伯数字,手写加减乘除号,手写小括号和手写等号。其中数据集中为手写阿拉伯数字,手写加减乘除号,手写小括号和手写等号的图像
时间: 2024-10-29 15:00:38 浏览: 5
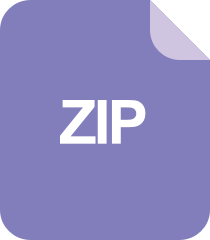
人工智能四则运算手写体识别训练模型
为了完成这个任务,我们将使用Python的深度学习库`TensorFlow`和`Keras`,以及`MNIST`数据集作为基础,因为`MNIST`包含了手写数字的样本。然而,由于你需要额外的手写字母和运算符的自定义数据,这里首先介绍如何使用`MNIST`作为模板,然后说明如何添加自定义数据。
首先安装所需的库:
```bash
pip install tensorflow numpy keras matplotlib
```
接下来,我们编写一个基本的`CNN`模型用于识别手写字符,并在最后添加自定义数据部分:
```python
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# 使用MNIST数据集
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.reshape(-1, 28, 28, 1).astype('float32') / 255.0
x_test = x_test.reshape(-1, 28, 28, 1).astype('float32') / 255.0
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
# 创建模型
model = Sequential([
Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(64, kernel_size=(3, 3), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dropout(0.5),
Dense(10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
# 添加自定义数据部分 - 这需要你自己提供数据和相应的标签
# 示例假设你已经有了两个numpy数组,分别是自定义数据`custom_images`和对应的标签`custom_labels`
# custom_images应调整到相同的大小(28x28,单通道)
# custom_labels需转换成one-hot编码
custom_images = ... # 自定义数据集
custom_labels = ... # 标签数据
# 预处理自定义数据
custom_images = custom_images.reshape(-1, 28, 28, 1).astype('float32') / 255.0
custom_labels = tf.keras.utils.to_categorical(custom_labels, num_classes=11) # 11类包括数字、运算符和特殊字符
# 将自定义数据合并到训练集
x_train = np.concatenate((x_train, custom_images))
y_train = np.concatenate((y_train, custom_labels))
# 更新模型的输入形状并继续训练
model.layers[0].input_shape = (None, 28, 28, 1)
model.fit(x_train, y_train, epochs=5, batch_size=64) # 调整epoch数和batch_size以适应新数据量
# 测试
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f"Test accuracy: {test_acc}")
# 相关问题--
1. 如何收集和标记自定义的手写字母和运算符数据?
2. 如何确保自定义数据的质量和一致性以便模型可以学习?
3. 如果自定义数据集很大,应该如何分批加载数据以避免内存溢出?
```
阅读全文
相关推荐
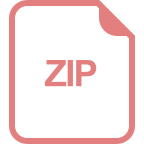
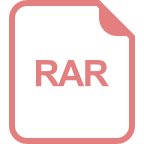
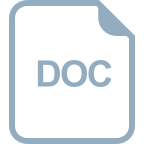
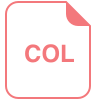













